Matplotlib – Scatter Plot with Random Values
In this tutorial, you’ll learn how to create a scatter plot using Matplotlib in Python, where the data points of the scatter plot are generated from NumPy random.rand().
A scatter plot is useful for visualizing the relationship between two sets of data points.
The following is a step by step tutorial on how to draw a Scatter Plot using Matplotlib.
1. Import Necessary Libraries
Begin by importing Matplotlib library.
import matplotlib.pyplot as plt
Optionally, you can also import NumPy for generating sample data.
import numpy as np
2. Generate Sample Data
Create two arrays of data points for the X and Y axes. For this example, we’ll use NumPy to generate random data.
# Number of data points
num_points = 50
# Generate random values for X and Y
x_values = np.random.rand(num_points)
y_values = np.random.rand(num_points)
3. Create Scatter Plot
Use Matplotlib’s scatter()
function to create a scatter plot with the generated data points.
# Create scatter plot
plt.scatter(x_values, y_values, label='Scatter Plot')
4. Customize and Show the Plot
Customize the plot by adding a title, labels for the X and Y axes, and a legend. Finally, display the plot using show()
.
# Customize the plot
plt.title('Scatter Plot Example')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
# Show the plot
plt.show()
Complete Program for Scatter Plot with Random Data Points
Here’s the complete program to draw a Scatter Plot using Matplotlib, where the data points are generated using NumPy random.rand() function.
Python Program
import matplotlib.pyplot as plt
import numpy as np
# Number of data points
num_points = 50
# Generate random values for X and Y
x_values = np.random.rand(num_points)
y_values = np.random.rand(num_points)
# Create scatter plot
plt.scatter(x_values, y_values, label='Scatter Plot')
# Customize the plot
plt.title('Scatter Plot Example')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
# Show the plot
plt.show()
Output
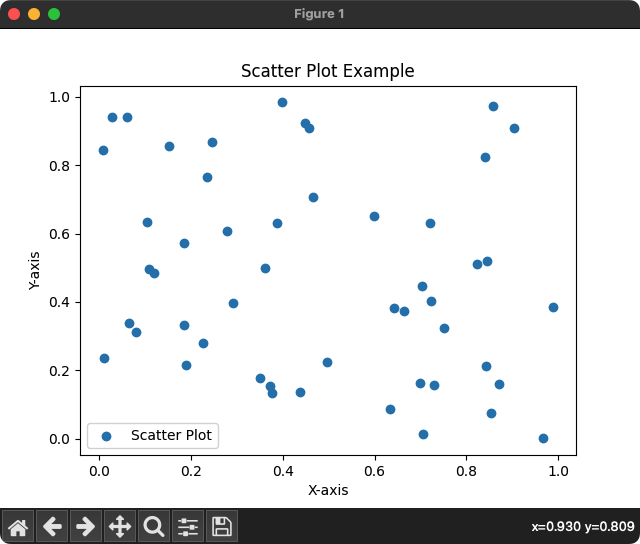
Summary
This tutorial covered the basics of creating a scatter plot using Matplotlib in Python.