Matplotlib – Scatter Plot Color
In this tutorial, we’ll explore how to customize the color of data points in a Matplotlib scatter plot. Adjusting the colors can enhance the visual representation of different categories or patterns in your data.
The following is a step by step process to customize the color for scatter plot in Matplotlib.
1. Import Necessary Libraries
Begin by importing the required Matplotlib library.
import matplotlib.pyplot as plt
Optionally, you can also import NumPy for generating sample data.
import numpy as np
2. Generate Sample Data
Create arrays of data points for the X and Y axes and an additional array for colors. For this example, we’ll use NumPy to generate random data and assign colors based on conditions.
# Number of data points
num_points = 50
# Generate random values for X and Y
x_values = np.random.rand(num_points)
y_values = np.random.rand(num_points)
3. Create Scatter Plot with Customized Color
Use Matplotlib’s scatter()
function to create a scatter plot with the generated data points and customized colors.
Pass the required color for the c
parameter of the scatter function. Let us say that we would like to display the points in 'red'
color.
# Create scatter plot with customized color
plt.scatter(x_values, y_values, c='red', label='Scatter Plot')
4. Customize and Show the Plot
Customize the plot by adding a title, labels for the X and Y axes, and a legend. Finally, display the plot using show()
.
# Customize the plot
plt.title('Scatter Plot with Customized Colors')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
# Show the plot
plt.show()
Complete Program for Scatter Plot with Custom Color
Let us put all the above mentioned steps together, and write a program for a scatter plot with ‘red’ color points.
Python Program
import matplotlib.pyplot as plt
import numpy as np
# Number of data points
num_points = 50
# Generate random values for X and Y
x_values = np.random.rand(num_points)
y_values = np.random.rand(num_points)
# Create scatter plot with customized color
plt.scatter(x_values, y_values, c='red', label='Scatter Plot 1')
# Customize the plot
plt.title('Scatter Plot with Customized Colors')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
# Show the plot
plt.show()
Output
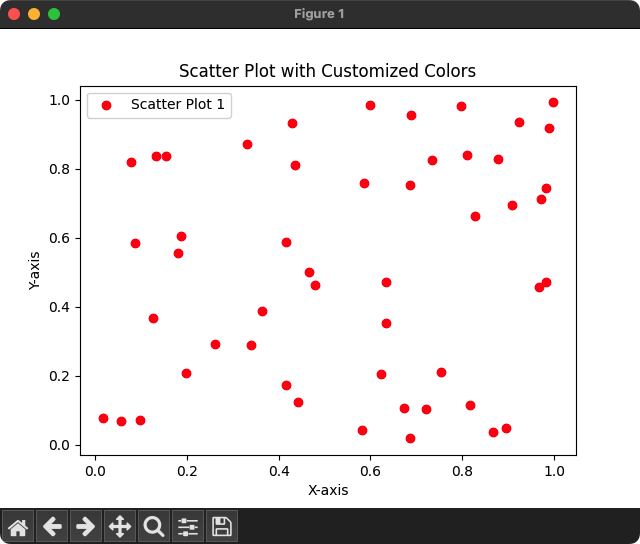
If there are multiple scatter plots in the same figure, and if we want to set different colors for different data sets, then we can set them as shown in the following program.
Python Program
import matplotlib.pyplot as plt
import numpy as np
# Number of data points
num_points = 50
# Generate random values for X and Y for both plots
x_values_1 = np.random.rand(num_points)
y_values_1 = np.random.rand(num_points)
x_values_2 = np.random.rand(num_points)
y_values_2 = np.random.rand(num_points)
# Create scatter plots for both datasets with custom color
plt.scatter(x_values_1, y_values_1, c='red', label='Scatter Plot 1')
plt.scatter(x_values_2, y_values_2, c='green', label='Scatter Plot 2')
# Customize the plot
plt.title('Two Scatter Plots Example')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
# Show the plot
plt.show()
Output
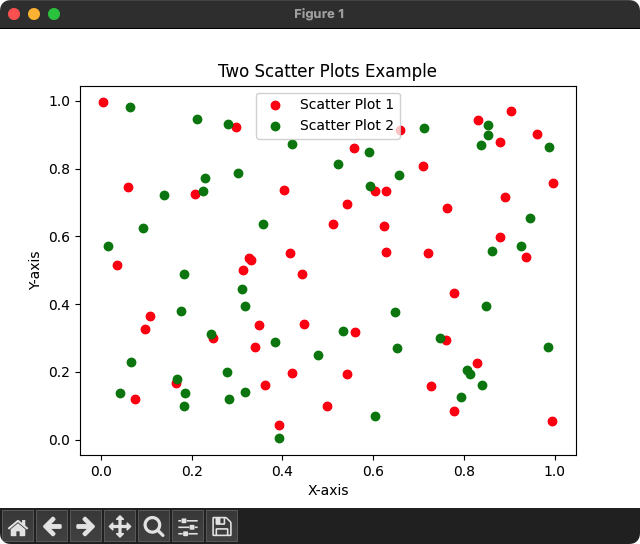
Summary
This tutorial demonstrated how to customize the color of data points in a Matplotlib scatter plot, providing a visual distinction between different categories or patterns within the data.