Matplotlib – Label Font Size
To set a specific font size for a label of the plot in Matplotlib, you can use fontsize
parameter, or fontdict
parameter of the label functions.
In this tutorial, we shall go through both the approaches, and look into the situations when to use which.
Approach 1: Using fontsize
parameter
This approach is used when you would like to change the font size for a specific label, when granular customization is required.
plt.xlabel('X-axis Sample Label', fontsize = 10)
plt.ylabel('Y-axis Sample Label', fontsize = 20)
X-axis label is set with a font size of 10, and Y-axis label is set with a font size of 20. You can also use other font size values depending on your preference.
Python Program
import matplotlib.pyplot as plt
# Example data
x = [1, 2, 3, 4, 5]
y = [10, 20, 35, 45, 30]
# Plot the data
plt.plot(x, y, marker='o')
# Set font size for the labels
plt.xlabel('X-axis Sample Label', fontsize = 10)
plt.ylabel('Y-axis Sample Label', fontsize = 20)
# Show the plot
plt.show()
Output
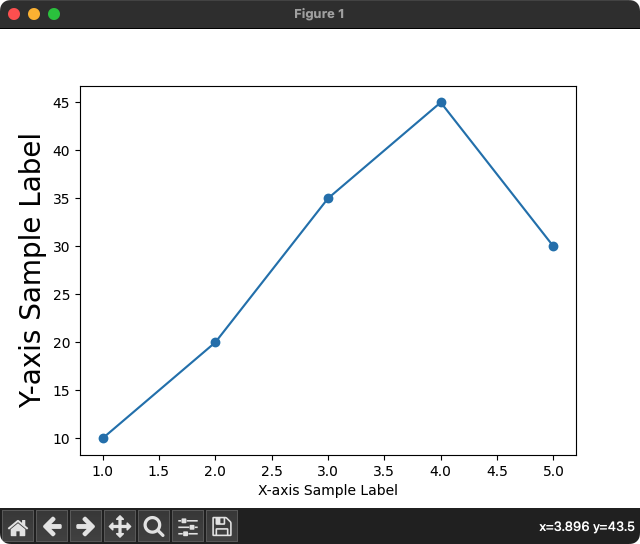
Approach 2: Using fontdict
parameter
This approach is useful when you would like to set a specific font for all the labels, like a global setting for the font, and you would like to make the code more modular. In the font dictionary object, specify the size attribute with the required size value.
You can also specify the font family, and font color in addition to the font size.
In the following program, we set the font size to 15 for X-axis and Y-axis labels.
Python Program
import matplotlib.pyplot as plt
# Example data
x = [1, 2, 3, 4, 5]
y = [10, 20, 35, 45, 30]
# Plot the data
plt.plot(x, y, marker='o')
# Specify font size
my_font = {'size': 15}
# Set fontdict for the labels
plt.xlabel('X-axis Sample Label', fontdict = my_font)
plt.ylabel('Y-axis Sample Label', fontdict = my_font)
# Show the plot
plt.show()
Output
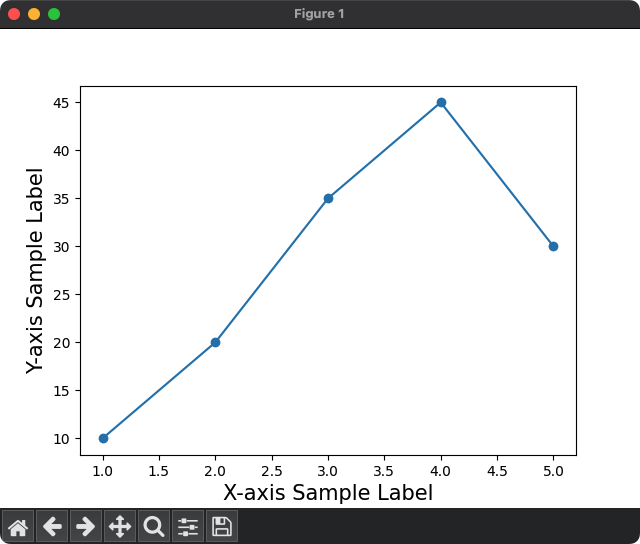