Matplotlib – Plot Line style
While drawing line plot, you can specify the style of the line using linestyle
parameter of the plot() function.
Solid Plot Line: plot(linestyle=’solid’)
To plot a solid line, specify linestyle='solid'
to the plot() function. By default, the line style is solid for the line plot. Therefore, even if you do not specify any value for linestyle, a solid line is drawn for line plot.
In the following program, we draw a solid plot line.
Python Program
import matplotlib.pyplot as plt
# Example data
x = [1, 2, 3, 4, 5]
y = [20, 30, 55, 70, 60]
# Plot solid line
plt.plot(x, y, linestyle='solid', marker='o')
# Customize plot
plt.title('Solid Line Plot Example')
plt.xlabel('X-axis Label')
plt.ylabel('Y-axis Label')
# Show the plot
plt.show()
Output
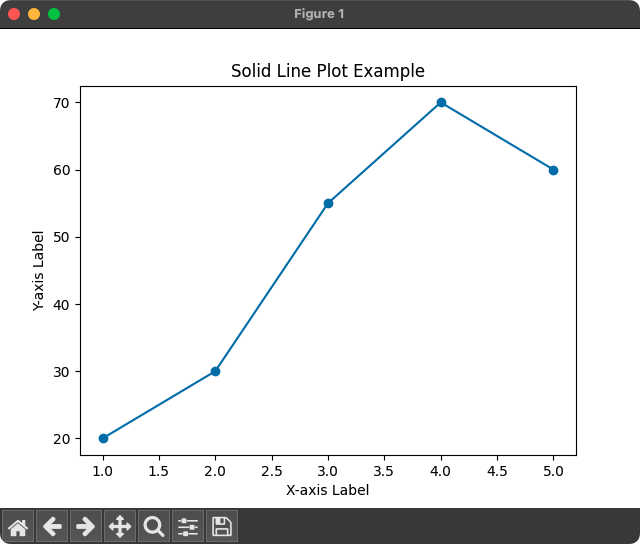
Dotted Plot Line: plot(linestyle=’dotted’)
To plot a solid line, specify linestyle='dotted'
to the plot() function.
In the following program, we plot a dotted plot line using Matplotlib.
Python Program
import matplotlib.pyplot as plt
# Example data
x = [1, 2, 3, 4, 5]
y = [20, 30, 50, 70, 60]
# Plot dotted line
plt.plot(x, y, linestyle='dotted', marker='o')
# Customize plot
plt.title('Dotted Line Plot Example')
plt.xlabel('X-axis Label')
plt.ylabel('Y-axis Label')
# Show the plot
plt.show()
Output
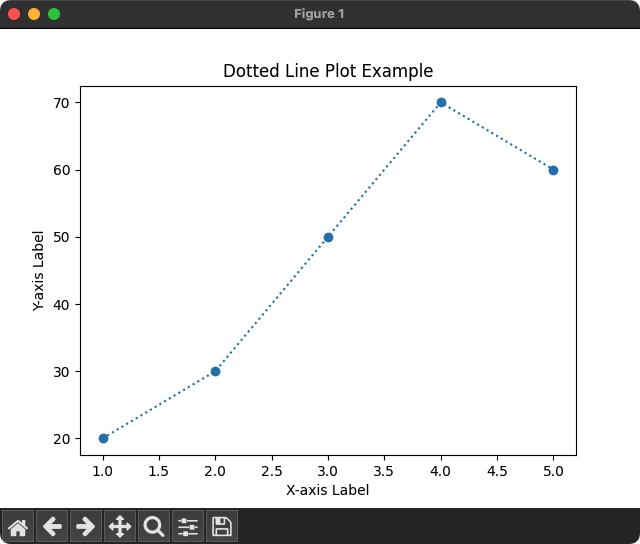
Dashed Plot Line: plot(linestyle=’dashed’)
To plot a solid line, specify linestyle='dashed'
to the plot() function.
In the following program, we draw a dashed plot line.
Python Program
import matplotlib.pyplot as plt
# Example data
x = [1, 2, 3, 4, 5]
y = [20, 30, 55, 70, 60]
# Plot dashed line
plt.plot(x, y, linestyle='dashed', marker='o')
# Customize plot
plt.title('Dashed Line Plot Example')
plt.xlabel('X-axis Label')
plt.ylabel('Y-axis Label')
# Show the plot
plt.show()
Output
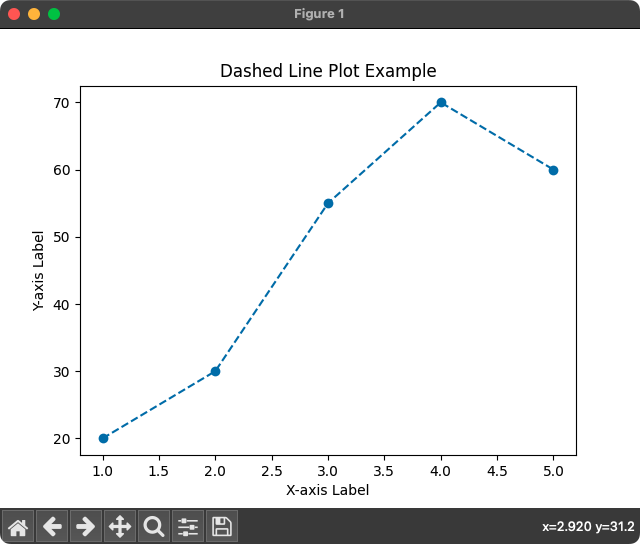
Dashdot Plot Line: plot(linestyle=’dashdot’)
To plot a dash-dot line, specify linestyle='dashdot'
to the plot() function.
In the following program, we draw a dashdot plot line.
Python Program
import matplotlib.pyplot as plt
# Example data
x = [1, 2, 3, 4, 5]
y = [20, 30, 55, 70, 60]
# Plot dashdot line
plt.plot(x, y, linestyle='dashdot', marker='o')
# Customize plot
plt.title('Dashdot Line Plot Example')
plt.xlabel('X-axis Label')
plt.ylabel('Y-axis Label')
# Show the plot
plt.show()
Output
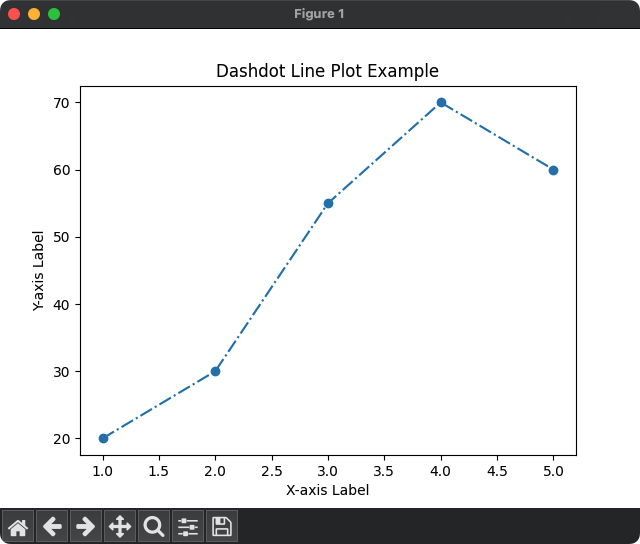
Summary
Concluding this tutorial, we have seen how to use linestyle parameter of plot() function to change the style of plot line.