Matplotlib – Subplot with two rows and two columns
First let us see the syntax of subplot() function, and then using this information, we shall create subplot with two rows and two columns.
The syntax of subplot function is
subplot(crows, cols, index, **kwargs)
where
Parameter | Description |
---|---|
nrows | Number of rows of subplots. |
ncols | Number of columns of subplots. |
index | Integer or tuple representing the index of the current subplot. Starts from 1. |
**kwargs | Additional keyword arguments passed to the `add_subplot` call (optional). |
Therefore, to create subplot with two rows and two columns,
- The first subplot should be
plt.subplot(2, 2, 1)
where the first argument2
specifies that there are two rows, the second argument2
specifies that there are two columns, and the third argument1
specifies that this subplot is the first. - The second subplot should be
plt.subplot(2, 2, 2)
where the first argument2
specifies that there are two rows, the second argument2
specifies that there are two columns, and the third argument2
specifies that this subplot is the second. - Similarly, the third subplot should be
plt.subplot(2, 2, 3)
where the third argument3
specifies that this subplot is the third. - And, the fourth subplot should be
plt.subplot(2, 2, 4)
where the third argument4
specifies that this subplot is the fourth.
For example, consider the following program where we create subplot with two rows and two columns.
Python Program
import matplotlib.pyplot as plt
# Example data
x1 = [1, 2, 3, 4, 5]
y1 = [10, 20, 25, 55, 40]
x2 = [1, 2, 3, 4, 5]
y2 = [10, 5, 15, 20, 40]
x3 = [1, 2, 3, 4, 5]
y3 = [0.8, 5.5, 1.5, 2.1, 0.7]
x4 = [1, 2, 3, 4, 5]
y4 = [4, 6, 5, 2, 4]
# Create a figure with two subplots (1 row, 2 columns)
plt.figure(figsize=(10, 4))
# Subplot 1
plt.subplot(2, 2, 1)
plt.plot(x1, y1)
plt.title('Data 1 Graph')
plt.xlabel('Data 1 X-axis')
plt.ylabel('Data 1 Y-axis')
# Subplot 2
plt.subplot(2, 2, 2)
plt.plot(x2, y2)
plt.title('Data 2 Graph')
plt.xlabel('Data 2 X-axis')
plt.ylabel('Data 2 Y-axis')
# Subplot 3
plt.subplot(2, 2, 3)
plt.plot(x3, y3)
plt.title('Data 3 Graph')
plt.xlabel('Data 3 X-axis')
plt.ylabel('Data 3 Y-axis')
# Subplot 4
plt.subplot(2, 2, 4)
plt.plot(x4, y4)
plt.title('Data 4 Graph')
plt.xlabel('Data 4 X-axis')
plt.ylabel('Data 4 Y-axis')
# Adjust layout for better spacing
plt.tight_layout()
# Show the plot
plt.show()
Output
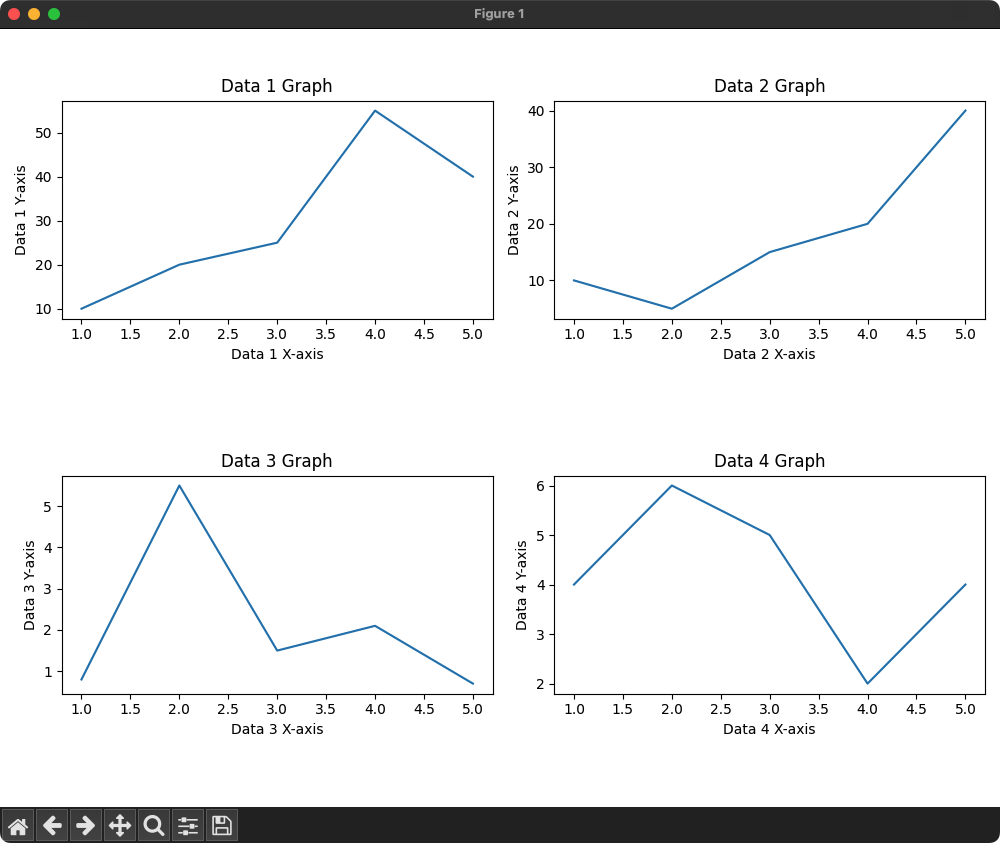