Matplotlib – Plot Multiple Lines Tutorial
In this tutorial, we’ll create a plot with multiple lines using Matplotlib in Python.
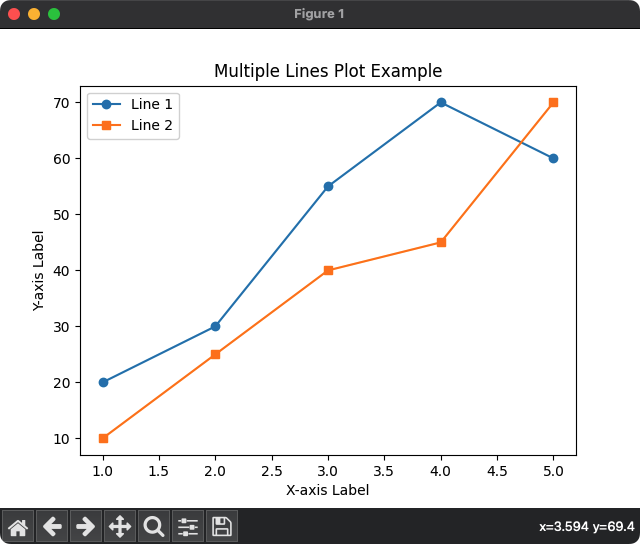
1. Import Matplotlib.pyplot
Import the Matplotlib library, specifically the pyplot
module.
import matplotlib.pyplot as plt
2. Create Data for Multiple Lines
Define the data points for the X-axis and multiple Y-axes.
x = [1, 2, 3, 4, 5]
y1 = [20, 30, 55, 70, 60]
y2 = [10, 25, 40, 55, 70]
In this case, x
represents the values on the X-axis, and y1
and y2
represent the corresponding values for two lines.
3. Plot Multiple Lines
Use Matplotlib to plot multiple lines on the same plot.
plt.plot(x, y1, label='Line 1', marker='o')
plt.plot(x, y2, label='Line 2', marker='s')
label
: This parameter provides a label for each line, which will be used in the legend.
For the first line we specified circular marker, while for the second line square shaped marker is specified.
4. Customize and Show the Plot
Customize the plot with labels, titles, and legends, and then show the plot in a figure.
plt.title('Multiple Lines Plot Example')
plt.xlabel('X-axis Label')
plt.ylabel('Y-axis Label')
plt.legend()
plt.show()
Complete program to plot Multiple Lines using Matplotlib
Using all the above steps, let us write the complete program to draw multiple lines using Matplotlib plot() function.
Python Program
import matplotlib.pyplot as plt
# Example data
x = [1, 2, 3, 4, 5]
y1 = [20, 30, 55, 70, 60]
y2 = [10, 25, 40, 45, 70]
# Plot multiple lines
plt.plot(x, y1, label='Line 1', marker='o')
plt.plot(x, y2, label='Line 2', marker='s')
# Customize plot
plt.title('Multiple Lines Plot Example')
plt.xlabel('X-axis Label')
plt.ylabel('Y-axis Label')
plt.legend()
# Show the plot
plt.show()
Output
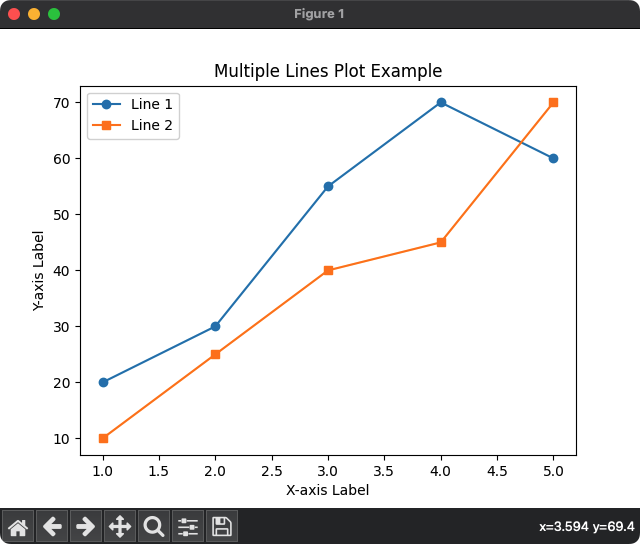
Summary
That concludes our tutorial on creating a plot with multiple lines using Matplotlib in Python!