Matplotlib – subplot
In Matplotlib, subplots enable you to create multiple plots within a single figure, allowing for side-by-side or grid-based visualizations.
For example, consider the following program where we create two subplots horizontally in a row.
Python Program
import matplotlib.pyplot as plt
# Example data
x = [1, 2, 3, 4, 5]
y1 = [10, 20, 25, 55, 40]
y2 = [10, 5, 15, 20, 40]
# Create a figure with two subplots (1 row, 2 columns)
plt.figure(figsize=(10, 4))
# Subplot 1 (left)
plt.subplot(1, 2, 1)
plt.plot(x, y1)
plt.title('Data 1 Graph')
plt.xlabel('Data 1 X-axis')
plt.ylabel('Data 1 Y-axis')
# Subplot 2 (right)
plt.subplot(1, 2, 2)
plt.plot(x, y2)
plt.title('Data 2 Graph')
plt.xlabel('Data 2 X-axis')
plt.ylabel('Data 2 Y-axis')
# Adjust layout for better spacing
plt.tight_layout()
# Show the plot
plt.show()
Output
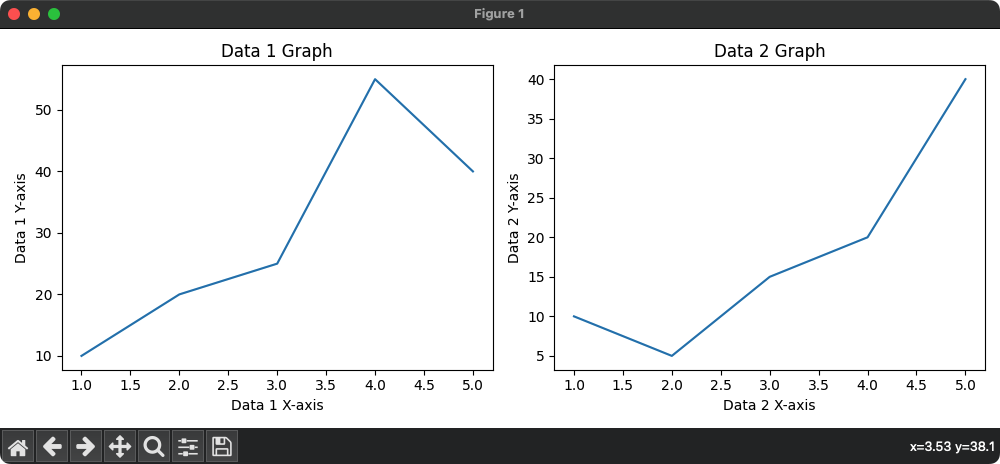
In this example
plt.subplot(1, 2, 1)
creates the first subplot on the left.plt.subplot(1, 2, 2)
creates the second subplot on the right.
The syntax of subplot function is
subplot(crows, cols, index, **kwargs)
where
Parameter | Description |
---|---|
nrows | Number of rows of subplots. |
ncols | Number of columns of subplots. |
index | Integer or tuple representing the index of the current subplot. Starts from 1. |
**kwargs | Additional keyword arguments passed to the `add_subplot` call (optional). |
In our example, the parameters (1, 2, 1)
and (1, 2, 2)
indicate that there is one row and two columns of subplots, and the third parameter specifies the index of the current subplot.