Tkinter Button Example
Python tkinter Button
When you are building a GUI application, button is one the building block that makes your application interactive.
In this tutorial, we shall learn how to implement Button in Python GUI using tkinter Python library.
Syntax to create Tkinter Button
The syntax to add a Button to the tkinter window is:
mybutton = Button(master, option=value)
mybutton.pack()
Where master is the window to which you would like to add this button. tkinter provides different options to the button constructor to alter its look.
Option | Value |
---|---|
text | The text displayed as Button Label |
width | To set the width of the button |
height | To set the height of the button |
bg | To set the background color of the button |
fg | To set the font color of the button label |
activebackground | To set the background color when button is clicked |
activeforeground | To set the font color of button label when button is clicked |
command | To call a function when the button is clicked |
font | To set the font size, style to the button label |
image | To set the image on the button |
Examples
1. Create Button in Tkinter
In the following example, we will create a tkinter button with the following properties.
text | "My Button" |
width | 40 |
height | 3 |
bg | '#0052cc' |
fg | '#ffffff' |
activebackground | '#0052cc' |
activeforeground | '#aaffaa' |
Python Program
from tkinter import *
gui = Tk(className='Python Examples - Button')
gui.geometry("500x200")
# create button
button = Button(gui, text='My Button', width=40, height=3, bg='#0052cc', fg='#ffffff', activebackground='#0052cc', activeforeground='#aaffaa')
# add button to gui window
button.pack()
gui.mainloop()
Explanation
- The
tkinter
library is imported, which is used for creating GUI applications in Python. - A Tk object
gui
is created, which represents the main window of the application. The window is given the title'Python Examples - Button'
, and its size is set to500x200
pixels using thegeometry()
method. - A
Button
widget is created with the following properties: text='My Button'
: Sets the text displayed on the button.width=40
,height=3
: Specifies the width and height of the button.bg='#0052cc'
,fg='#ffffff'
: Sets the background and foreground colors of the button.activebackground='#0052cc'
,activeforeground='#aaffaa'
: Defines the button's colors when it is clicked (active state).- The
button.pack()
method is used to add the button to the window and position it. - Finally,
gui.mainloop()
is called to start the tkinter event loop, allowing the window to be displayed and interactive.
Output
When you run this python program, you will get the following Window.
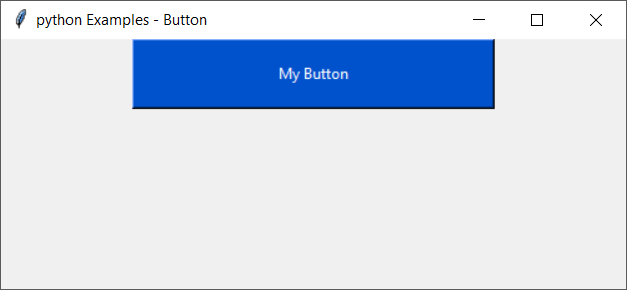
Summary
In this tutorial of Python Examples, we learned how to create a GUI button in Python using tkinter.