Contents
Tkinter Label font size
To set the font size of a Label widget in Tkinter, create a Font object with desired font size by setting size parameter with required integer value, and pass this font object as argument to the font parameter of Label() constructor.
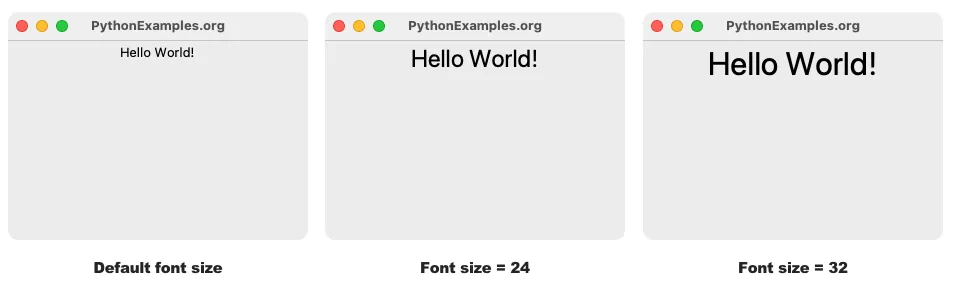
In this tutorial, you will learn how to set the font size of text in a Label widget, with examples.
Steps to set specific font size for Label in Tkinter
Step 1
Import font from tkinter library, and create a Font class object with required size.
from tkinter import font
label_font = font.Font(size=24)
Step 2
Create a label widget using tkinter.Label class constructor and pass the label_font as argument to the font parameter.
tk.Label(window, text="Hello World!", font=label_font)
Examples
1. Label with font size=24
In this example, we shall create a Label with text Hello World and font size of 24, and display it in the main window.
Program
import tkinter as tk
from tkinter import font
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
# Create font object
label_font = font.Font(size=24)
# Create a label widget with specific font
label = tk.Label(window, text="Hello World!", font=label_font)
# Pack the label widget to display it
label.pack()
# Run the application
window.mainloop()
Output
Run on a MacOS.
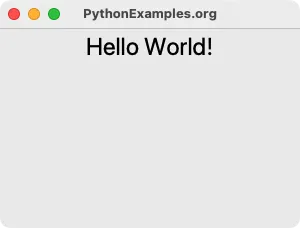
2. Label with font size=32
Now, let us change the font size to 32 and display the Label.
Program
import tkinter as tk
from tkinter import font
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
# Create a font object
label_font = font.Font(size=24)
# Create a label widget with specific font
label = tk.Label(window, text="Hello World!", font=label_font)
# Pack the label widget to display it
label.pack()
# Run the application
window.mainloop()
Output
Run on a MacOS.
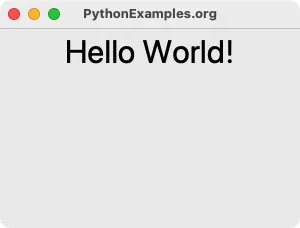
Summary
In this Python Tkinter tutorial, we learned how to create a Label widget with specific font size, with examples.