Tkinter Entry - On Change Event Handler
Tkinter Entry - On Change
In Tkinter, you can set a function to execute whenever the value in Entry widget changes.
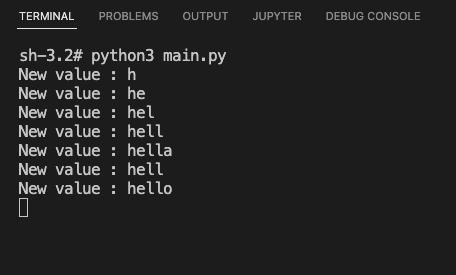
To set an on-change event handler function for the content of a Tkinter Entry widget, you can use the StringVar
class along with the trace()
method.
For example, the following code creates a StringVar to store the value entered in an Entry widget.
entry_var = tk.StringVar()
Then call trace() method on the StringVar object and pass "w" and the on change event handler function as arguments.
entry_var.trace("w", on_entry_change)
on_entry_change function is called whenever there is a change in the value of Entry widget.
In this tutorial, you will learn how to handle the on change event for the value in Entry widget, with examples.
Examples
1. Print value in Entry widget on change in value
In this example, we create a basic Tk window with an Entry widget. Wherever the value changes in the Entry widget by the user of programmatically, we print the value to standard output.
Python Program
import tkinter as tk
# Function to handle the Entry widget value change
def on_entry_change(*args):
new_value = entry_var.get()
print("New value :", new_value)
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
label = tk.Label(window, text="Enter input")
label.pack()
# Create a StringVar to track the Entry widget value
entry_var = tk.StringVar()
# Set the trace method to call on_entry_change() when the value changes
entry_var.trace("w", on_entry_change)
# Create an Entry widget
entry = tk.Entry(window, textvariable=entry_var)
entry.pack()
# Run the application
window.mainloop()
Output
In Windows
In MacOS
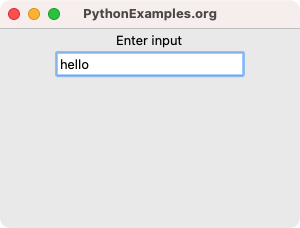
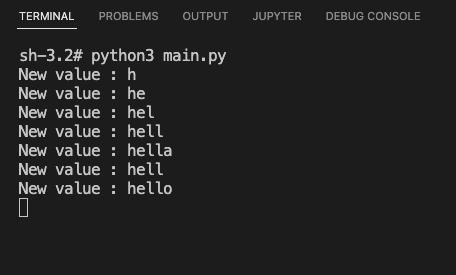
Summary
In this Python Tkinter tutorial, we have seen how to set on-change event handler for Entry widget in Tkinter, with examples.