Tkinter – Insert text into Entry widget programmatically
In Tkinter, you can enter a specific text into an Entry widget programmatically using Entry.insert() method.
To insert some text into an Entry field in Tkinter, call the insert() method and specify the index and value as arguments.
insert(index, value)
where
- index is the position at which the value is inserted
- value is the text which is inserted in the Entry field
You can give index=0 to insert the value at beginning of the Entry field.
Or, you can given index=tk.END to insert the value at ending of the Entry field, if the Entry field has any prior value.
In this tutorial, you will learn how to insert a given value at specified index in the Tkinter Entry widget, with examples.
Examples
1. Insert value at beginning of the Entry field
In this example, we create a basic Tk window with an Entry widget and button. You may enter some input in the field, or not. Then click on the Insert button. When user clicks the button, insert() method is used insert a value “Hello World “ at the start of the Entry field.
Python Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
label = tk.Label(window, text="Enter input value")
label.pack()
# Function to insert value at beginning in Entry field
def insert_value_in_entry():
entry.insert(0, "Hello World ")
# Create an Entry field
entry = tk.Entry(window)
entry.pack()
# Create a button
button = tk.Button(window, text="Insert", command=insert_value_in_entry)
button.pack()
# Run the application
window.mainloop()
Output
In Windows
In MacOS
Run the program.
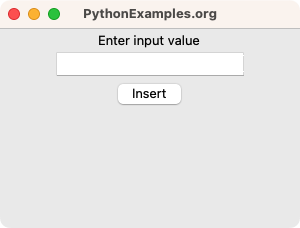
You may enter a value, and then click on Insert button, or just click on the Insert button without entering a value.
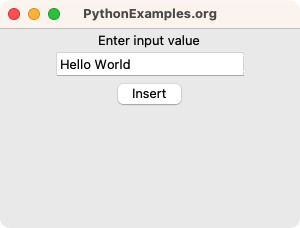
The specified value is inserted at the beginning of the Entry field.
2. Insert value at ending of the Entry field
In this example, we create a basic Tk window with an Entry widget and button. After entering the input in the Entry field, the user clicks on the Insert button. At the click, insert() method inserts a value ” Hello World” at the end of the Entry field.
Python Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
label = tk.Label(window, text="Enter input value")
label.pack()
# Function to insert value at end in Entry field
def insert_value_in_entry():
entry.insert(tk.END, " Hello World")
# Create an Entry field
entry = tk.Entry(window)
entry.pack()
# Create a button
button = tk.Button(window, text="Insert", command=insert_value_in_entry)
button.pack()
# Run the application
window.mainloop()
Output
In Windows
In MacOS
Run the program, enter a value in the field.
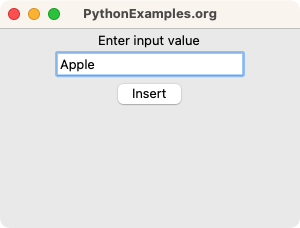
And click on Submit button.
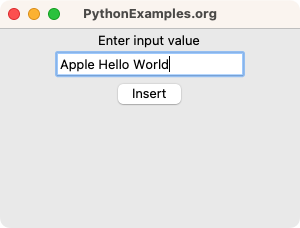
On clicking Insert button, the function insert_value_in_entry() is called, and it inserts ” Hello World” at the end in the Entry field.
Similarly, you can insert a value at any index, say index=2, index=5, etc., based on the length of the existing value.
Summary
In this Python Tkinter tutorial, we learned how to insert a value in the Entry field in Tkinter, with examples.