Tkinter Checkbutton – Command
Tkinter Checkbutton command option is used to set a callback function. The command or callback function is called when the Checkbutton is selected or unselected.
We have to define this command or callback function prior to using it for the Checkbutton command option.
Now, let us see how we can set this command option for the Checkbutton widget.
We can set a command function while creating the Checkbutton as shown below.
checkbutton_1 = tk.Checkbutton(window, text="Option 1", variable=checkbutton_1_var, command=checkbutton_toggle)
We have set the command option for the Checkbutton with the checkbutton_toggle function, as we already know, we have to define this function. And please be noted, that this function takes no arguments.
You can also set the command option with a function after creating the Checkbutton as shown in the following.
checkbutton_1 = tk.Checkbutton(window, text="Option 1", variable=checkbutton_1_var)
checkbutton_1['command'] = checkbutton_toggle
In this tutorial, you shall learn how to set the command option for a Checkbutton in Tkinter, with examples.
Example
In this example, we shall create a Checkbutton with command option set to the function checkbutton_toggle. Inside this function, we shall print if the Checkbutton is selected or deselected.
Python Program
import tkinter as tk
def set_values():
checkbutton_1_var.set(0)
checkbutton_2_var.set(1)
checkbutton_3_var.set(1)
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
# Variables for Checkbuttons
checkbutton_1_var = tk.IntVar()
checkbutton_2_var = tk.IntVar()
checkbutton_3_var = tk.IntVar()
# Create Checkbuttons in a loop
checkbutton_1 = tk.Checkbutton(window, text="Option 1", variable=checkbutton_1_var)
checkbutton_2 = tk.Checkbutton(window, text="Option 2", variable=checkbutton_2_var)
checkbutton_3 = tk.Checkbutton(window, text="Option 3", variable=checkbutton_3_var)
checkbutton_1.pack()
checkbutton_2.pack()
checkbutton_3.pack()
button = tk.Button(window, text="Set values", command=set_values)
button.pack()
# Start the Tkinter event loop
window.mainloop()
CopyOutput in MacOS
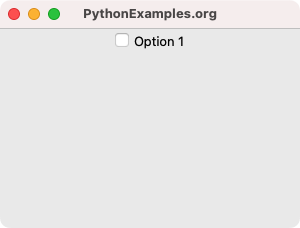
User clicks on the Checkbutton.
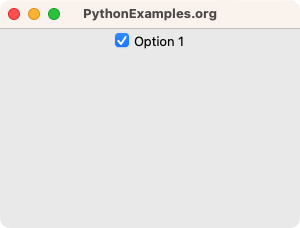
The command function is called.
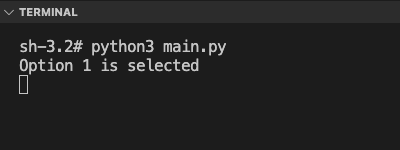
Summary
In this Python Tkinter tutorial, we have seen how to set command option for the Checkbutton widget in Tkinter, with examples.