Tkinter – Get text values of selected Checkbuttons
To get the text values of all the selected Checkbutton widgets in Tkinter, get the variables associated with the Checkbuttons and find those that are selected.
For example, consider that we created Checkbutton widgets using a dictionary, like the one shown in the following.
options = {
"Option 1": tk.IntVar(),
"Option 2": tk.IntVar(),
"Option 3": tk.IntVar()
}
Now, in the callback function of an event in which you would like to get the selected values, use a For loop statement as shown in the following.
selected_options = []
for option, var in options.items():
if var.get() == 1:
selected_options.append(option)
In the For loop statement, we iterate over the variables of the Checkbutton widgets, and collect the values of the selected Checkbuttons in a list selected_options.
In this tutorial, you shall learn how to get the text values of selected Checkbuttons in Tkinter, with examples.
Example
In this example, we shall create three Checkbuttons using a dictionary, and a button Show Selected. When user clicks on the Show Selected button, we print the text values of the selected Checkbuttons.
Python Program
import tkinter as tk
def show_selected():
selected_options = []
for text, var in options.items():
if var.get() == 1:
selected_options.append(text)
print("Selected options:", selected_options)
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
# Text and Variable for Checkbuttons
options = {
"Option 1": tk.IntVar(),
"Option 2": tk.IntVar(),
"Option 3": tk.IntVar()
}
# Create Checkbuttons in a loop
for option, var in options.items():
check_button = tk.Checkbutton(root, text=option, variable=var)
check_button.pack(anchor=tk.W)
button = tk.Button(root, text="Show Selected", command=show_selected)
button.pack()
# Start the Tkinter event loop
window.mainloop()
CopyOutput in MacOS
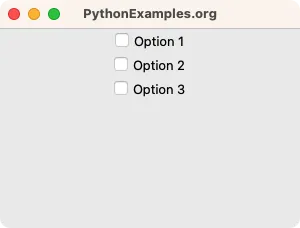
User selects Option 2.
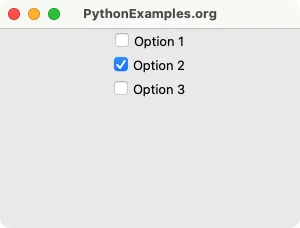
The callback function show_selected executes, and prints the list of selected options.
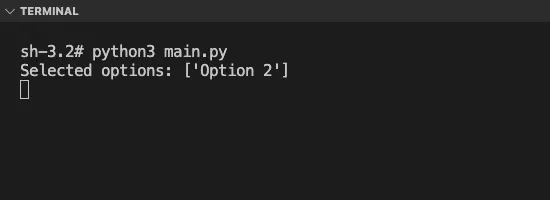
User selects another option, Option 3.
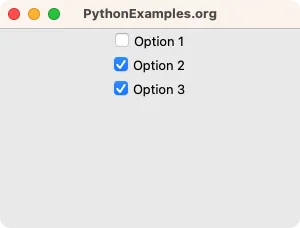
The callback function show_selected executes, and prints the list of selected options.
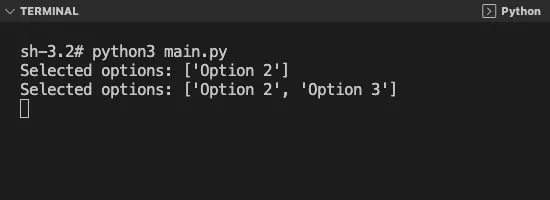
Summary
In this Python Tkinter tutorial, we have seen how to get the text values of selected Checkbutton widgets in Tkinter, with examples.