How to change Tkinter Button font style? Examples
Python Tkinter Button - Change Font Style
You can change the font properties like font-family, font size, font weight, etc., of Tkinter Button, by using tkinter.font package. In your Python program, import tkinter.font as font, create font.Font() object with required options and assign the Font object to font option of Button.
In this tutorial, we shall learn how to change the font-family, font size and font weight, with the help of well detailed example Python programs.
Pseudo Code - Change Button Font
Following is the pseudo code to change font style of Tkinter Button.
import tkinter.font as font
#create Font object
myFont = font.Font(family='Helvetica')
button = Button(parent, font=myFont)
#or
button = Button(parent)
button['font'] = myFont
Examples
1. Change font family of Button to Helvetica
In this example, we will change the font family of tkinter button using family named argument provided to font.Font().
Python Program
from tkinter import *
import tkinter.font as font
gui = Tk(className='Python Examples - Button')
gui.geometry("500x200")
# define font
myFont = font.Font(family='Helvetica')
# create button
button = Button(gui, text='My Button', bg='#0052cc', fg='#ffffff')
# apply font to the button label
button['font'] = myFont
# add button to gui window
button.pack()
gui.mainloop()
Explanation
- The
tkinter
library is imported for creating the GUI and thefont
module is imported to define a custom font for the button. - A Tk object
gui
is created, representing the main window of the application. The window is titled'Python Examples - Button'
. - The window size is set to
500x200
pixels using thegeometry()
method. - A custom font
myFont
is defined usingfont.Font(family='Helvetica')
, which sets the font to Helvetica. - A button widget is created with the text
'My Button'
, a background color#0052cc
, and a foreground color#ffffff
. - The defined font
myFont
is applied to the button using thebutton['font'] = myFont
statement. - The button is then added to the GUI window using
button.pack()
, and the main loop of the tkinter application is started withgui.mainloop()
.
Output
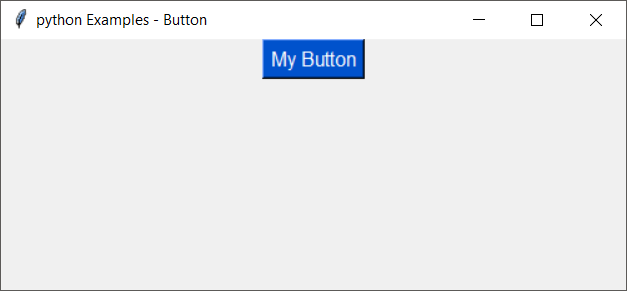
Without font, the button would look like in the following GUI window.
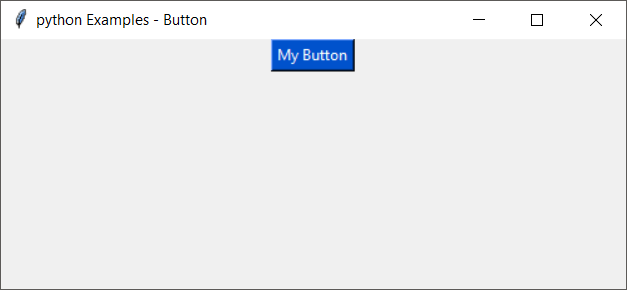
You can compare the output of our next examples with this button and find how a font property has affected the button.
2. Change font size of tkinter Button to 30
You can also change font size of the text in tkinter Button, by passing named argument size
to font.Font().
In this example, we will change the font size of tkinter button.
Python Program
from tkinter import *
import tkinter.font as font
gui = Tk(className='Python Examples - Button')
gui.geometry("500x200")
# define font
myFont = font.Font(size=30)
# create button
button = Button(gui, text='My Button', bg='#0052cc', fg='#ffffff')
# apply font to the button label
button['font'] = myFont
# add button to gui window
button.pack()
gui.mainloop()
Explanation
- The
tkinter
library is imported for creating the GUI, and thefont
module is imported to define a custom font for the button. - A Tk object
gui
is created to represent the main window of the application. The window is titled'Python Examples - Button'
. - The window size is set to
500x200
pixels using thegeometry()
method. - A custom font
myFont
is defined with a font size of30
usingfont.Font(size=30)
. - A button widget is created with the text
'My Button'
, a background color of#0052cc
, and a foreground color of#ffffff
. - The defined font
myFont
is applied to the button usingbutton['font'] = myFont
. - The button is then added to the GUI window using
button.pack()
, and the main loop of the tkinter application is started withgui.mainloop()
.
Output
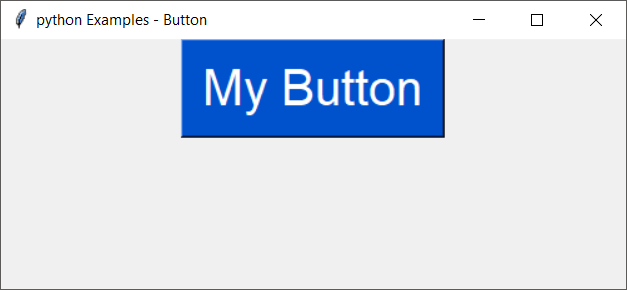
Font size of the button is 30.
3. Change font weight of tkinter Button to bold
You can change font weight of the text in tkinter Button, by passing named argument weight
to font.Font().
In this example, we will change the font weight of tkinter button.
Python Program
from tkinter import *
import tkinter.font as font
gui = Tk(className='Python Examples - Button')
gui.geometry("500x200")
# define font
myFont = font.Font(weight="bold")
# create button
button = Button(gui, text='My Button', bg='#0052cc', fg='#ffffff')
# apply font to the button label
button['font'] = myFont
# add button to gui window
button.pack()
gui.mainloop()
Explanation
- The
tkinter
library is imported for creating the GUI, and thefont
module is imported to define a custom font for the button. - A Tk object
gui
is created to represent the main window of the application. The window is titled'Python Examples - Button'
. - The window size is set to
500x200
pixels using thegeometry()
method. - A custom font
myFont
is defined with a bold weight usingfont.Font(weight="bold")
. - A button widget is created with the text
'My Button'
, a background color of#0052cc
, and a foreground color of#ffffff
. - The defined font
myFont
is applied to the button usingbutton['font'] = myFont
. - The button is then added to the GUI window using
button.pack()
, and the main loop of the tkinter application is started withgui.mainloop()
.
Output
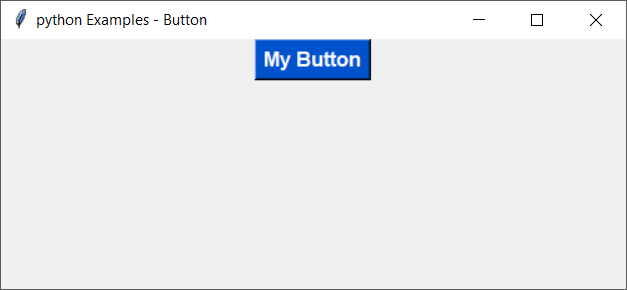
4. Change font family, size, and style of Button in a single statement.
We can apply all the font styling together with font.Font().
In this example, we will change font family, font size and font weight.
Python Program
from tkinter import *
import tkinter.font as font
gui = Tk(className='Python Examples - Button')
gui.geometry("500x200")
# define font
myFont = font.Font(family='Helvetica', size=20, weight='bold')
# create button
button = Button(gui, text='My Button', bg='#0052cc', fg='#ffffff')
# apply font to the button label
button['font'] = myFont
# add button to gui window
button.pack()
gui.mainloop()
Explanation
- The
tkinter
library is imported for creating the GUI, and thefont
module is imported to define a custom font for the button. - A Tk object
gui
is created to represent the main window of the application. The window is titled'Python Examples - Button'
. - The window size is set to
500x200
pixels using thegeometry()
method. - A custom font
myFont
is defined with the following properties:family='Helvetica'
sets the font family to Helvetica.size=20
sets the font size to 20.weight='bold'
makes the font bold.
- A button widget is created with the text
'My Button'
, a background color of#0052cc
, and a foreground color of#ffffff
. - The defined font
myFont
is applied to the button usingbutton['font'] = myFont
. - The button is then added to the GUI window using
button.pack()
, and the main loop of the tkinter application is started withgui.mainloop()
.
Output
When you run this application, you will get the window
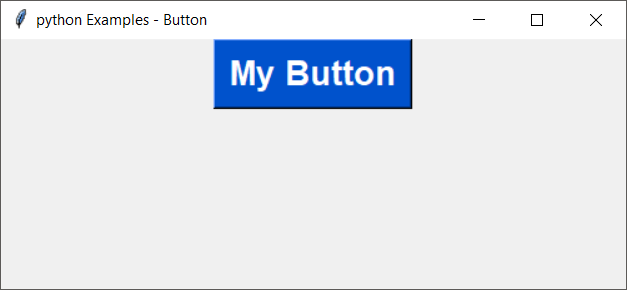
Let us change the font family to Courier and run the application.
myFont = font.Font(family='Courier', size=20, weight='bold')
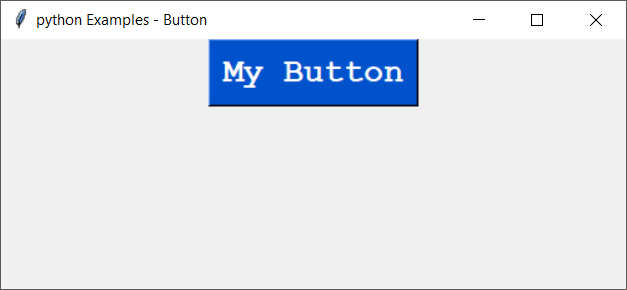
Summary
In this tutorial of Python Examples, we changed the font family, font size and font weight of tkinter Button, with the help of well detailed examples.