Tkinter Create Menu
To create a menu in Tkinter, use Tk.Menu class.
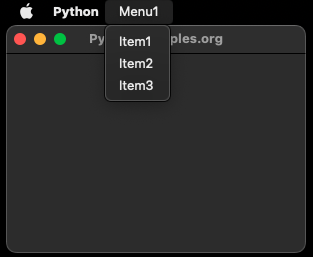
Let us consider that we need to build a window using Tkinter, with the following menu.
Menu1 # File is a menu in menu bar
Item1 # item under file menu
Item2 # item under file menu
Item3 # item under file menu
When user clicks on the Menu1 menu, the items in the menu are displayed.
Steps to create Menu using Tkinter
Step 1
Import tkinter, and create a Tk object.
import tkinter as tk
window = tk.Tk()
Step 2
Create Menu bar using Tk.Menu class.
menu_bar = tk.Menu(window)
Step 3
Create a Menu in the Menu bar using Tk.Menu class.
menu_1 = tk.Menu(menu_bar, tearoff=0)
menu_bar is the master of menu_1.
Step 4
Add items to the menu. There are different kinds of items that can be added to a menu. Examples are command items, cascade items, checkbutton items, radio button items, etc.
In this example, we shall add command items to the menu.
menu_1.add_command(label="Item1")
menu_1.add_command(label="Item2")
menu_1.add_command(label="Item3")
We have added three command items to the menu_1. For all these three command items, menu_1 is the master.
Step 5
Add menu to the menu bar. That is, add File menu menu_1 to the main Menu menu_bar.
menu_bar.add_cascade(label="MyMenu", menu=menu_1)
We have labelled the menu as Menu1. The label Menu1 would be displayed for the menu in the Menu bar.
Step 6
Attach the menu bar to the main Tk window.
window.config(menu=menu_bar)
Step 7
Start the Tkinter event loop.
window.mainloop()
Example
The following is the complete program by combining all the above steps to create and display a menu in the window.
Python Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
# Create the menu bar
menu_bar = tk.Menu(window)
# Create the Menu1 menu
menu_1 = tk.Menu(menu_bar, tearoff=0)
menu_1.add_command(label="Item1")
menu_1.add_command(label="Item2")
menu_1.add_command(label="Item3")
# Add Menu1 to the menu bar
menu_bar.add_cascade(label="Menu1", menu=menu_1)
# Attach the menu bar to the main window
window.config(menu=menu_bar)
# Start the Tkinter event loop
window.mainloop()
Output on MacOS
Run the program.
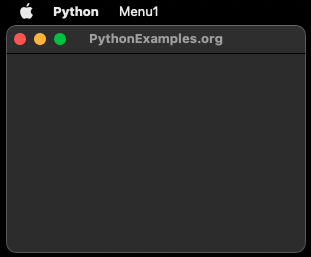
Hover on the Menu1.
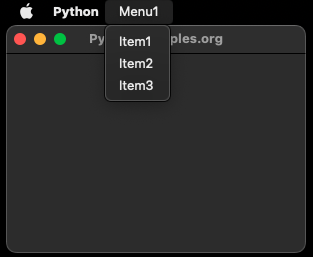
Summary
In this Python Tkinter tutorial, we learned how to create a Menu using Tkinter, with the help of examples.