Tkinter Checkbutton – Check if selected
We can check if a Checkbutton widget is selected or not in Tkinter using the variable option.
To check if a specific Checkbutton widget is selected or not in Tkinter, get the value in the Checkbutton variable option, and check if it is 1 or 0. If the value in the variable is 1, then the Checkbutton has been selected, or if the value in the variable is 0, then the Checkbutton has not been selected.
For example, in the following code snippet, where we have created a Checkbutton checkbutton_1 with variable checkbutton_var.
checkbutton_var = tk.IntVar()
checkbutton_1 = tk.Checkbutton(window, text="Option 1", variable=checkbutton_var)
We can set an event handler function for the state change of the Checkbutton, or some other event like clicking on a Button widget for submitting information, and in that event handler function, we can write the following logic.
if checkbutton_var.get():
print("Option 1 is selected")
else:
print("Option 1 is not selected")
In this tutorial, you will learn how to check if a Checkbutton is selected or not, with examples.
Example
In the following GUI application, we shall create a Checkbutton widget and a button. When user clicks on the button, we shall check if the Checkbutton is selected or not, and print the result to output.
You may replace the print statement with whatever logic required for your application in the if and else blocks.
Python Program
import tkinter as tk
def verify_checkbutton():
if checkbutton_var.get():
print("Option 1 is selected")
else:
print("Option 1 is not selected")
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
# Create a variable to store the checkbutton state
checkbutton_var = tk.IntVar()
# Create the checkbutton widget
checkbutton_1 = tk.Checkbutton(window, text="Option 1", variable=checkbutton_var)
checkbutton_1.pack()
# Button
mybutton = tk.Button(window, text="Submit", command=verify_checkbutton)
mybutton.pack()
# Start the Tkinter event loop
window.mainloop()
CopyOutput in MacOS
Run the application.
Click on the Submit button, without checking the option.
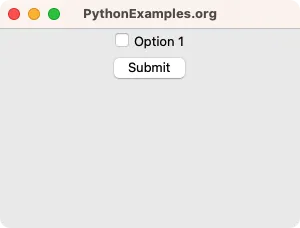
The following would be the output. Since the Checkbutton is not selected, checkbutton_var.get() returns 0, and the else-block is run.
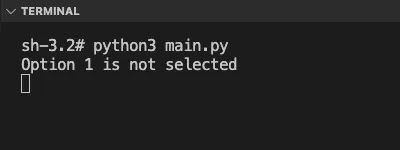
Run the application.
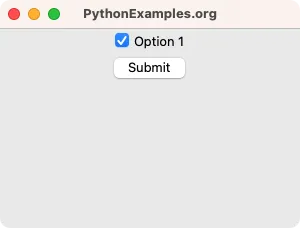
Since the Checkbutton is selected, checkbutton_var.get() returns 1, and the if-block is run.
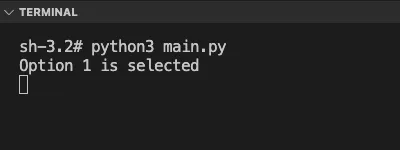
Summary
In this Python Tkinter tutorial, we have seen how to check if a Checkbutton widget is selected or not, with examples.