Tkinter Entry - Placeholder
Tkinter - Placeholder for Entry widget
In Tkinter, there is no support for placeholder by default. You can add a placeholder with some additional code.
To display a placeholder in an Entry widget, we define two functions. The first function is called when the Entry widget gets focus. The second function is called when the Entry widget is out of focus.
When the Entry widget has no focus, the display a value in the Entry widget, the placeholder value, with color of the text set to gray.
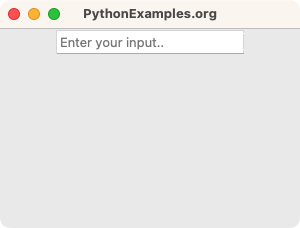
When the Entry widget has no focus, and if the text in the Entry widget is equal to the placeholder value, then we shall empty the Entry field, and set the text color to black.
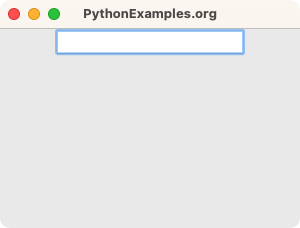
And then the user enter the input value.
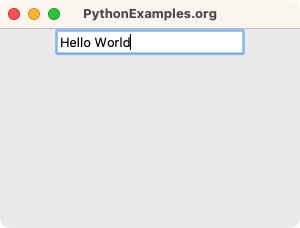
In this tutorial, you will learn how to add a placeholder to an Entry widget in Tkinter, with examples.
Examples
1. Add placeholder to Entry widget
In this example, we create a basic Tk window with an Entry widget. We shall define those two functions we mentioned above. Then we shall bind the Entry widget with the focus-in and focus-out events, and add the respective functions as event handler functions. This is how we add a Placeholder to the Entry widget.
Python Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
# Function to handle the Entry widget focus events
def on_entry_focus_in(event):
if entry.get() == placeholder_text:
entry.delete(0, tk.END)
entry.configure(show="")
entry.configure(fg="black")
def on_entry_focus_out(event):
if entry.get() == "":
entry.insert(0, placeholder_text)
entry.configure(show="*")
entry.configure(fg="gray")
# Create an Entry widget with placeholder text
placeholder_text = "Enter your input.."
entry = tk.Entry(window, fg="gray")
entry.insert(0, placeholder_text)
entry.bind("<FocusIn>", on_entry_focus_in)
entry.bind("<FocusOut>", on_entry_focus_out)
entry.pack()
# Run the application
window.mainloop()
Output
In Windows
In MacOS
Run the program. The Entry field is displayed with Placeholder.
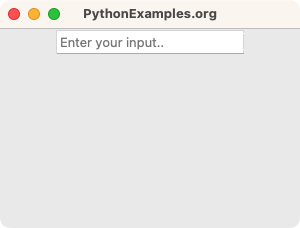
Click on the Entry widget. The focus-in event is triggered.
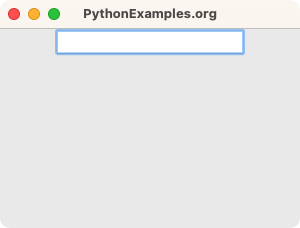
You may enter some value into the Entry widget.
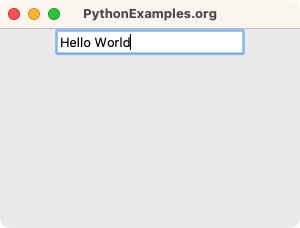
Summary
In this Python Tkinter tutorial, we have shown how to add a Placeholder to an Entry field in Tkinter, with examples.