Pass arguments in the command function for Menu item in Tkinter
To pass arguments in the callback function when user clicks on a menu item in Tkinter, wrap the command function in a lambda function.
For example, consider that we are adding an item Item_1 to the menu. We would like to set item_action as callback function for the menu item, and also pass an argument “Item 1” to the callback function.
The syntax to wrap the function with arguments in a lambda function and assign it to the command parameter is
command=lambda: item_action("Item 1")
In this tutorial, you will learn how to call a callback command function with arguments passed to it, when user clicks on a menu item.
Example
In this example, we shall create a menu with two menu items. When user clicks on the first item Item_1, we call item_action(“Item 1”). Similarly, when user clicks on the second item Item_2, we call item_action(“Item 2”). We are setting the same function item_action() as callback function for the menu items, but the argument we pass changes with the menu item.
Program
import tkinter as tk
def item_action(value):
print(f'You clicked on {value}')
# Create the main window
window = tk.Tk()
# Create the menu bar
menu_bar = tk.Menu(window)
# Create the menu
my_menu = tk.Menu(menu_bar, tearoff=0)
# Add items for MyMenu1
my_menu.add_command(label="Item_1", command=lambda: item_action("Item 1"))
my_menu.add_command(label="Item_2", command=lambda: item_action("Item 2"))
# Add the menu to the menu bar
menu_bar.add_cascade(label="MyMenu", menu=my_menu)
# Attach the menu bar to the main window
window.config(menu=menu_bar)
# Start the Tkinter event loop
window.mainloop()
Output
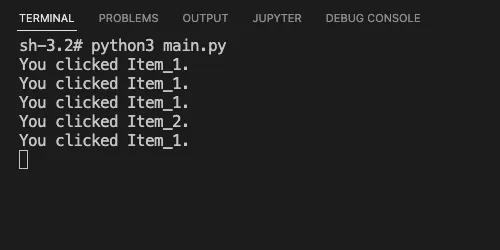
Summary
In this Python Tkinter tutorial, we learned how to pass arguments to the callback command function for a menu item, with the help of examples.