Tkinter – Get value from Entry widget
In Tkinter, Entry widget is used to read an input from the user. Once the user has entered a value, we can read that value using Entry.get() method.
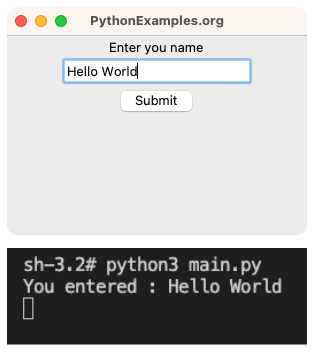
To get the value entered in an Entry field in Tkinter, call the get() method on the Entry object. The method returns a string value.
entry = tk.Entry(window)
entry.get()
Usually the value from the Entry field is read after an event like clicking a button, or submitting the values for validation, etc.
In this tutorial, you will learn how to get the value entered in a Tkinter Entry widget, with examples.
Examples
1. Get name of the user from Entry widget
In this example, we create a basic Tk window with an Entry field for entering the name of the user, and a button to submit the input value. When user clicks the button, we shall get the value entered in the Entry field, and display it in the console output.
Python Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
# Function to read and print the value in Entry widget
def print_entered_value():
value = entry.get()
print("You entered :", value)
label = tk.Label(window, text="Enter you name")
label.pack()
# Create an Entry field
entry = tk.Entry(window)
entry.pack()
# Create a button
button = tk.Button(window, text="Submit", command=print_entered_value)
button.pack()
# Run the application
window.mainloop()
Output
In Windows
In MacOS
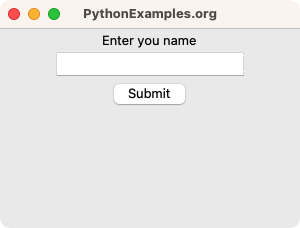
Enter a value in the entry filed, and click on Submit button.
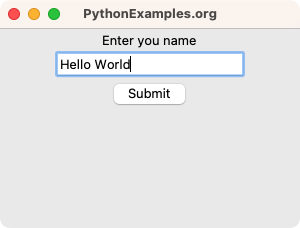
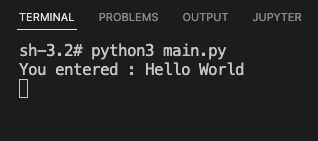
Summary
In this Python Tkinter tutorial, we learned how to get the value entered in an Entry filed in Tkinter, with examples.