Tkinter Entry - Handle Focus-In and Focus-Out events
Tkinter Entry - Handle Focus-In and Focus-Out Events
In Tkinter, you can handle the focus events of an Entry widget by binding the Entry widget to the Focus-In and Focus-Out events with respective event handler functions.
Focus-In event is triggered when the Entry widget gains focus.
Focus-Out event is triggered when the Entry widget loses focus.
We can bind these Focus-In and Focus-Out events to the Entry widget with respective event handler functions.
For example, consider the following code where we have an Entry widget entry. FocusIn event for the Entry widget is handled by the entry_focus_in function, and the FocusOut event is handled by the entry_focus_out function.
entry.bind("<FocusIn>", entry_focus_in)
entry.bind("<FocusOut>", entry_focus_out)
In this tutorial, you will learn how to bind FocusIn and FocusOut events to an Entry widget, with examples.
Examples
1. Bind Focus-In and Focus-Out events to Entry Widget
In this example, we create a basic Tk window with an Entry widget entry. We have bind the FocusIn and FocusOut events to the entry widget with the event handling functions entry_focus_in and entry_focus_out respectively.
Python Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
label = tk.Label(window, text="Enter input")
label.pack()
# Function to handle the Entry widget focus events
def entry_focus_in(event):
print('Entry got focus.')
def entry_focus_out(event):
print('Entry lost focus.')
# Create an Entry widget for username
entry = tk.Entry(window)
entry.bind("<FocusIn>", entry_focus_in)
entry.bind("<FocusOut>", entry_focus_out)
entry.pack()
# Run the application
window.mainloop()
Output
In Windows
In MacOS
Run the program.
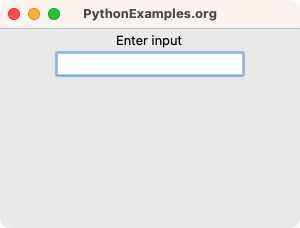
Click on the Entry widget to gain focus for the first time. Then click outside the window to lose focus. After that if you click on the window, the Entry regains its focus. And so on.
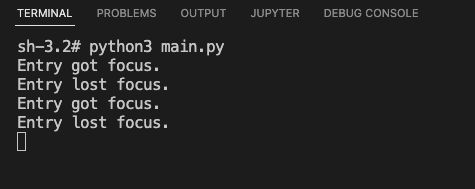
Summary
In this Python Tkinter tutorial, we have seen how to bind FocusIn and FocusOut events to an Entry widget in Tkinter, with examples.