How to set Tkinter Window Size? Examples
Python Tkinter - Set Window Size
Usually, when you create a gui using Tkinter, the size of the window depends on the size and placement of components in the window. But, you can also control the window size by setting a specific width and height to the window.
To set the size of Tkinter window, call geometry() function on the window with width and height string passed as argument.
In this tutorial, we shall learn how to set a specific window size to our Tkinter GUI application in Python, using example programs.
Syntax of geometry() method
To set a specific size to the window when using Python tkinter, use geometry() function on the Tk() class variable.
#import statement
from tkinter import *
#create GUI Tk() variable
gui = Tk()
#set window size
gui.geometry("widthxheight")
where width and height should be replaced with integers that represent the width and height of the window respectively. Observe that there is x
between width and height in the argument provided to geometry()
.
Note: Please note that window size width x height does not include the window title.
Examples
In the following examples, we shall see how to set the window size to specific dimensions.
1. Set window size to 500x200 in Tkinter
In this example, we will use geometry() method to set a fixed window size of 500 by 200 to the Tk() window.
Python Program
from tkinter import *
gui = Tk(className='Python Examples - Window Size')
# set window size
gui.geometry("500x200")
gui.mainloop()
Explanation
- The
tkinter
library is imported, which is used for creating GUI applications in Python. - A Tk object
gui
is created, representing the main window of the application. The window is given the title'Python Examples - Window Size'
. - The window size is set to
500x200
pixels using thegeometry()
method, which takes a string parameter specifying the width and height of the window. - The
gui.mainloop()
method is called to start the tkinter event loop, allowing the window to be displayed and interactive.
When you run this program, the following GUI window will open, with the window size specified in geometry()
- width of 500 and height of 200.
Output in Windows
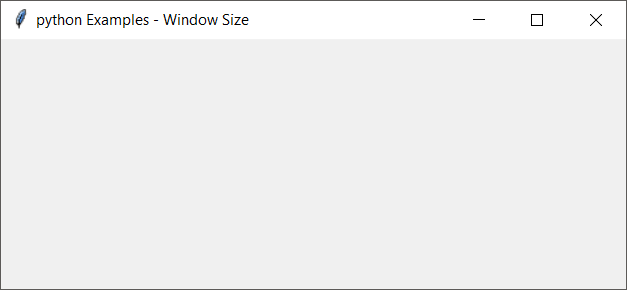
Output in macOS
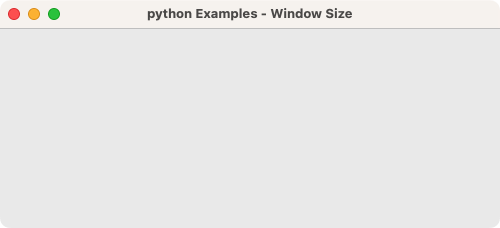
2. Set window size to 300x300 for your GUI application
Now, let us change the width and height provided to geometry() function. Say 300 by 300.
Python Program
from tkinter import *
gui = Tk(className='Python Examples - Window Size')
# set window size
gui.geometry("300x300")
gui.mainloop()
Explanation
- The
tkinter
library is imported, which is used for creating GUI applications in Python. - A Tk object
gui
is created, representing the main window of the application. The window is given the title'Python Examples - Window Size'
. - The window size is set to
300x300
pixels using thegeometry()
method, which takes a string parameter specifying the width and height of the window. - The
gui.mainloop()
method is called to start the tkinter event loop, allowing the window to be displayed and interactive.
Output in Windows
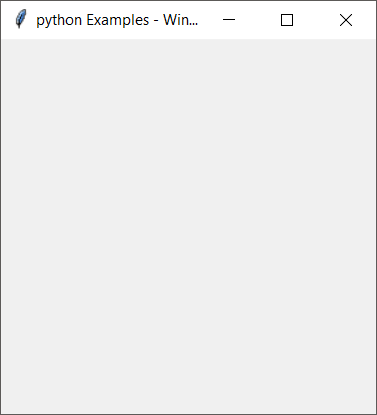
Output in macOS
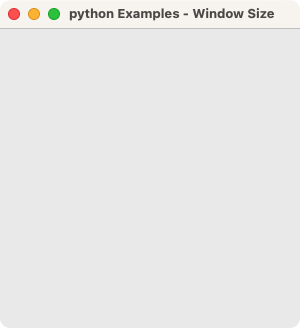
Summary
In this tutorial of Python Examples, we learned how to set the window size for a GUI application built using tkinter, with the help of well detailed examples.