Radiobutton Menu Items in Tkinter
In Tkinter, you can create menu items with Radio button functionality using the add_radiobutton() method of the Menu
class.
Radiobutton menu items allow users to select a single option from given set of radiobuttons.
To add a radiobutton menu item to a menu in Tkinter, call add_radiobutton()
method on the menu, and specify the values for label, variable, value, and command parameters.
add_radiobutton(label="Some text", variable=some_var, value="Some value" command=some_function)
- label is used to display the given text for the checkbox menu item.
- The given variable for variable parameter is used to store the value of the selected radiobutton. The variable has to be created prior to calling
add_radiobutton()
method. - The value given to the value parameter is the value of this radiobutton. This value would be assigned to the variable when this radiobutton is selected.
- The given function for command parameter is the callback function. This function is called whenever the state of the radiobutton is changed.
In this tutorial, you will learn how to add radiobutton menu items to a menu, with examples.
Steps to create Radiobutton Menu Items in Tkinter
Step 1
Define a function that can be used for the callback function of Radiobutton menu item.
def option_selected():
print("Selected option:", selected_option.get())
Inside the callback function, we retrieve the value of the option selected.
Please note that we create the variable selected_option later in step 3.
Step 2
Create a menu options_menu in menu bar using Tk.Menu class.
menu_bar = tk.Menu(window)
options_menu = tk.Menu(menu_bar, tearoff=False)
Step 3
Create a Tk boolean variable to store the value of selected radiobutton menu item.
selected_option = tk.StringVar()
Step 4
Add radiobutton menu items to the menu.
options_menu.add_radiobutton(label="Option 1", variable=selected_option, value="Option 1", command=option_selected)
options_menu.add_radiobutton(label="Option 2", variable=selected_option, value="Option 2", command=option_selected)
options_menu.add_radiobutton(label="Option 3", variable=selected_option, value="Option 3", command=option_selected)
Step 5
Cascade menu options_menu to the menu bar, and configure the Tk window with the menu bar.
Example
In this example, we shall create a menu options_menu in the menu bar. To this options_menu, we add three radiobutton menu items. User can select only a single radiobutton, by clicking the required radiobutton from the menu.
When user selects a radiobutton, the callback function is called, and we print the value of the selected radiobutton. You may use this value to know which radiobutton is selected, and implement your logic.
Program
import tkinter as tk
# Callback function for Radiobutton items in menu
def option_selected():
print("Selected option :", selected_option.get())
# Create the main window
window = tk.Tk()
# Create the menu bar
menu_bar = tk.Menu(window)
# Create a variable to store the state of the radiobutton
option_1 = tk.BooleanVar()
# Create a variable to store the selected option
selected_option = tk.StringVar()
# Create the "Options" menu
options_menu = tk.Menu(menu_bar, tearoff=0)
options_menu.add_radiobutton(label="Option 1", variable=selected_option, value="Option 1", command=option_selected)
options_menu.add_radiobutton(label="Option 2", variable=selected_option, value="Option 2", command=option_selected)
options_menu.add_radiobutton(label="Option 3", variable=selected_option, value="Option 3", command=option_selected)
# Add the menu to the menu bar
menu_bar.add_cascade(label="Options", menu=options_menu)
# Attach the menu bar to the main window
window.config(menu=menu_bar)
# Start the Tkinter event loop
window.mainloop()
Output
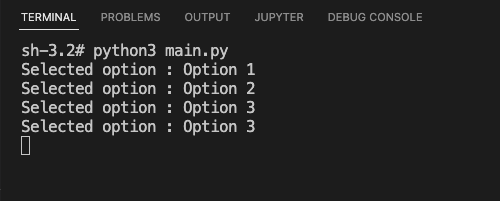
Summary
In this Python Tkinter tutorial, we learned how to add radiobutton menu items to a menu, with examples.