Tkinter - Create Checkbuttons from a dictionary
Tkinter - Create Checkbuttons from a dictionary
To create a set of Checkbutton widgets from a given dictionary in Tkinter, where each item in the dictionary has key for the text value of the Checkbutton widget, and value for the variable for the Checkbutton widget.
For example, consider the following dictionary.
options = {
"Option 1": tk.IntVar(),
"Option 2": tk.IntVar(),
"Option 3": tk.IntVar()
}
The first Checkbutton will have the text option set to "Option 1", and the variable option set to tk.IntVar().
Now, we shall write a Python program, where we create three Checkbutton widgets from the three key-value pairs of the given dictionary.
We shall assign the same callback function show_selected to all the three Checkbutton widgets. When there is a change in the selection, we shall print the selected options.
Also, we shall use a For loop statement to iterate over the items of the given dictionary.
Python Program
import tkinter as tk
def show_selected():
selected_options = []
for option, value in options.items():
if value.get() == 1:
selected_options.append(option)
print("Selected options:", selected_options)
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
options = {
"Option 1": tk.IntVar(),
"Option 2": tk.IntVar(),
"Option 3": tk.IntVar()
}
for option, value in options.items():
check_button = tk.Checkbutton(window, text=option, variable=value, command=show_selected)
check_button.pack()
window.mainloop()
Output in MacOS
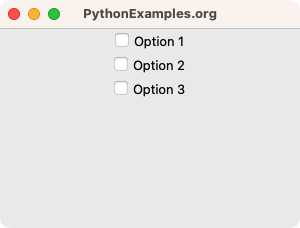
User selects Option 2.
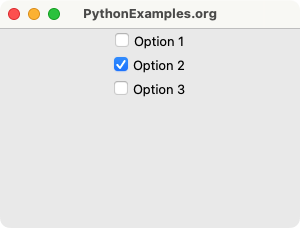
The callback function show_selected executes, and prints the list of selected options.
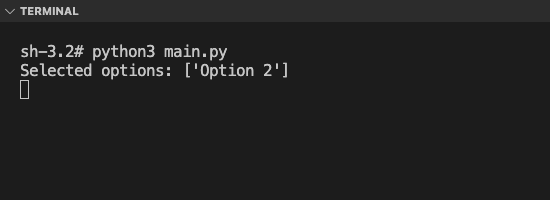
User selects another option, Option 3.
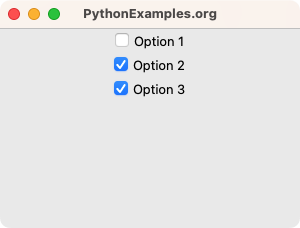
The callback function show_selected executes, and prints the list of selected options.
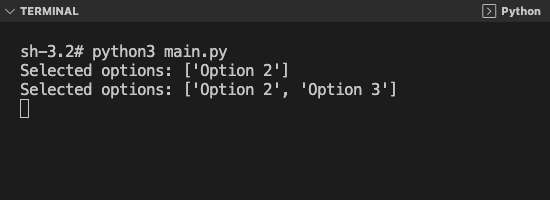
Summary
In this Python Tkinter tutorial, we have seen how to create Checkbutton widgets from the items of a dictionary, with examples.