Contents
Tkinter Entry – Focus next widget on enter key event
In Tkinter, you can move the focus from an Entry widget to the next widget on Enter key or Return key event.
To focus next widget on Enter key event in an Entry widget in Tkinter, bind the Entry widget with Return key and specify the event handler function using Entry.bind() method, as shown below.
entry_1.bind('<Return>', go_to_next_element)
Now, inside the event handler function go_to_next_element, take the focus to the next element.
def go_to_next_element(event):
event.widget.tk_focusNext().focus()
In this tutorial, you will learn how to take focus to the next widget on Enter/Return key event in Entry widget, with examples.
Examples
1. Take focus to next widget on Enter key in Entry widget
In this example, we create a basic Tk window with two Entry widgets. We bind the Return key event to the first Entry widget. If the user clicks Enter or Return key while entering a value in first Entry widget, we shall move the focus to the next element.
Python Program
import tkinter as tk
def go_to_next_element(event):
event.widget.tk_focusNext().focus()
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
label = tk.Label(window, text="Enter first input")
label.pack()
# First Entry widget
entry_1 = tk.Entry(window)
entry_1.bind('<Return>', go_to_next_element)
entry_1.pack()
label = tk.Label(window, text="Enter second input")
label.pack()
# Second Entry widget
entry_2 = tk.Entry(window)
entry_2.bind('<Return>', go_to_next_element)
entry_2.pack()
# Run the application
window.mainloop()
Output in Windows
Output in MacOS
Run the program.
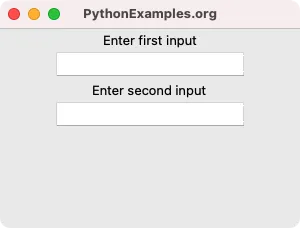
Enter some value, and press Enter/Return key.
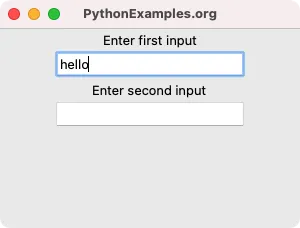
The event handler function takes the focus to the next element.
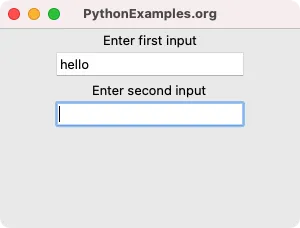
Summary
In this Python Tkinter tutorial, we have seen how to take focus to the next widget on Enter/Return key event in Entry widget in Tkinter, with examples.