Tkinter – Handle Window Resize Event
In Tkinter, you can handle the window resize event, and execute an event handler function whenever window resizes.
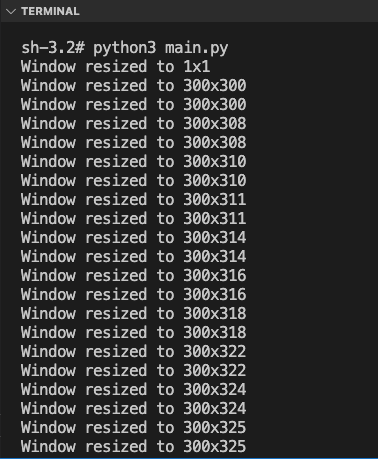
To handle the window resize event in Tkinter, bind the <Configure> event to the Tk window, and specify the event handler function.
window.bind("<Configure>", on_window_resize)
where
- window is the Tk window object.
- on_window_resize is the event handler function.
And the event handler function is as shown in the following.
def on_window_resize(event):
width = event.width
height = event.height
print(f"Window resized to {width}x{height}")
As in the above code, we can access the width and height of the window through event object.
Please note that the <Configure> event is triggered when the configuration of the window changes, like change in the width, height, position, etc.
In this tutorial, you will learn how to handle the window resize event in Tkinter with an event handler function using bind() method, with an example program.
Example
Consider a simple use case where you have a Tk window, and you would like to print the updated width and height of the window in the standard console output whenever the window resize happens.
Python Program
import tkinter as tk
from tkinter import font
def on_window_resize(event):
width = event.width
height = event.height
print(f"Window resized to {width}x{height}")
# Create the main window
window = tk.Tk()
window.geometry("300x300")
window.title("PythonExamples.org")
# Bind the resize event to a function
window.bind("<Configure>", on_window_resize)
# Run the application
window.mainloop()
Output in Windows
Output in MacOS
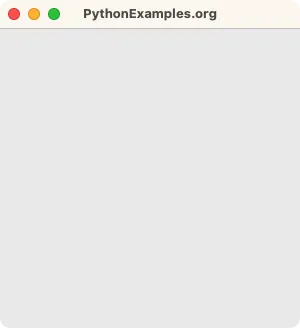
Try to resize the window, and you would see the updated size printed to output in the Terminal, as shown in the following screenshot.
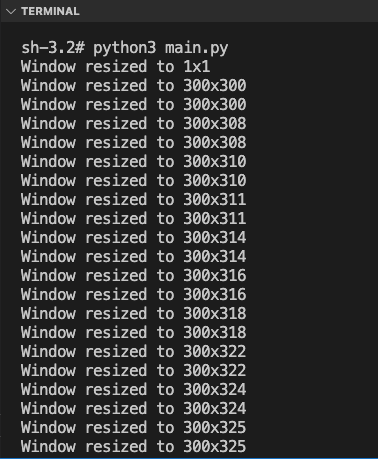
Summary
In this Python Tkinter tutorial, we learned how to handle the window resize event in Tkinter, with examples.