Tkinter Window - Resizable
Tkinter Window - Resizable
In Tkinter, you can specify whether user can resize the window or not, using resizable() method.
The syntax of resizable() method is
resizable(width, height)
where width and height are boolean values that specify whether the window can be resized along horizontal or vertical axis, respectively.
To create a resizable window using Tkinter, call resizable() method on the main window (Tk object), and pass True for both width and height options.
window = tk.Tk()
window.resizable(True, True)
To create a resizable window only along the width (horizontal axis), call resizable() method on the main window (Tk object), and pass True for the width option, and False for the height option.
window = tk.Tk()
window.resizable(True, False)
To create a resizable window only along the height (vertical axis), call resizable() method on the main window (Tk object), and pass False for the width option, and True for the height option.
window = tk.Tk()
window.resizable(True, False)
To create a non-resizable window in Tkinter, call resizable() method on the main window (Tk object), and pass False for the both width and height options.
window = tk.Tk()
window.resizable(False, False)
Now, let us go through some examples.
Examples
1. Create a resizable window in Tkinter
In this example, we shall create a resizable Tkinter window.
Python Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
# Set windows as resizable
window.resizable(True, True)
window.mainloop()
Output in MacOS
2. Set only the width of window as resizable
In this example, we shall create a Tkinter window that can be resized only along width.
Python Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
# Set windows as resizable only along width
window.resizable(True, False)
window.mainloop()
Output in MacOS
3. Set only the height of window as resizable
In this example, we shall create a Tkinter window that can be resized only along height.
Python Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
# Set windows as resizable only along height
window.resizable(False, True)
window.mainloop()
Output in MacOS
4. Non resizable window in Tkinter
In this example, we shall create a Tkinter window that cannot be resized along width or height.
Python Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
# Set windows as non-resizable
window.resizable(False, False)
window.mainloop()
Output in MacOS
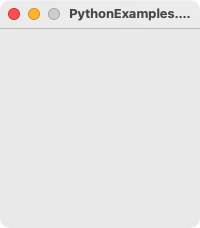
This window cannot the resized. Besides resizing, if you observe the window controls, only the minimize and close buttons of the window are active, and the maximize button is inactive.
Summary
In this Python Tkinter tutorial, we have seen how to set a window as resizable or not using resizable() method in Tkinter, with examples.