Tkinter Entry - Clear/Delete value in the field
Tkinter - Clear/Delete value in the Entry field
In Tkinter, you can clear the contents of an Entry field using Entry.delete() method.
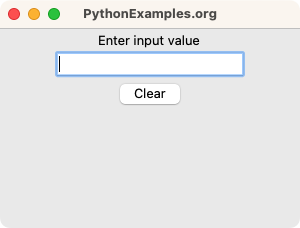
To clear all the contents of an Entry field in Tkinter, call the delete() method on the Entry object and specify the first and last indices.
delete(0 ,'end')
where
- 0 specifies the first index in the Entry widget
- 'end' specifies the last index in the Entry widget
In this tutorial, you will learn how to delete or clear all the text in an Entry field in Tkinter, with examples.
Examples
1. Insert value at beginning of the Entry field
In this example, we create a basic Tk window with an Entry widget and button. You may enter some input in the Entry field. Then click on the Clear button. When you click the button, delete() method is used to clear the Entry field.
Python Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
label = tk.Label(window, text="Enter input value")
label.pack()
# Function to clear the Entry field
def clear_entry():
entry.delete(0, 'end')
# Create an Entry field
entry = tk.Entry(window)
entry.pack()
# Create a button
button = tk.Button(window, text="Clear", command=clear_entry)
button.pack()
# Run the application
window.mainloop()
Output
In Windows
In MacOS
Run the program and enter some value in the Entry field.
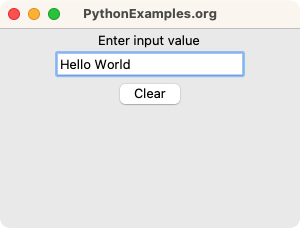
Click on the Clear button.
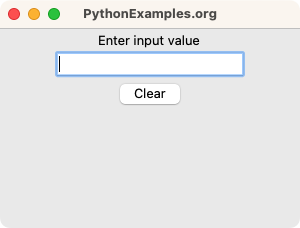
Summary
In this Python Tkinter tutorial, we have shown how to clear or delete a value in an Entry field in Tkinter, with examples.