Contents
Tkinter Label – Border
There is no default option to specify a border for Label widget in Tkinter. But, we can wrap this Label widget in a Frame, and specify border for the Frame widget.
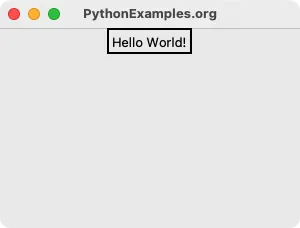
To set the border for a Label widget in Tkinter, wrap the Label widget in a Frame widget, and set the border for the Frame widget.
frame = tk.Frame(window, relief=tk.SOLID, borderwidth=2)
label = tk.Label(frame, text="Hello, Tkinter!")
In this tutorial, you will learn how to set the border for a Label widget using Frame widget, with examples.
Examples
1. Set SOLID border of 2px around the Label widget using Frame
In this example, we shall create a Frame widget with a solid border width of 2, and then use this Frame widget as a parent for the Label widget.
Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
# Create a frame with a border
frame = tk.Frame(window, relief=tk.SOLID, borderwidth=2)
# Create a label widget inside the frame
label = tk.Label(frame, text="Hello World!")
# Pack the label widget to display it
label.pack()
# Pack the frame to display it
frame.pack()
# Run the application
window.mainloop()
Output
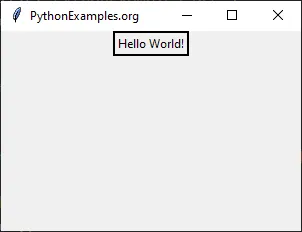
Run on a MacOS.
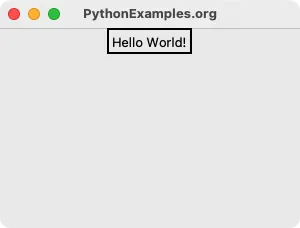
2. Set RAISED border of 5px around the Label widget using Frame
In this example, we shall create a Frame widget with a raised border with width of 5, and then use this Frame widget as a parent for the Label widget.
Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
# Create a frame with a border
frame = tk.Frame(window, relief=tk.RAISED, borderwidth=5)
# Create a label widget inside the frame
label = tk.Label(frame, text="Hello World!")
# Pack the label widget to display it
label.pack()
# Pack the frame to display it
frame.pack()
# Run the application
window.mainloop()
Output
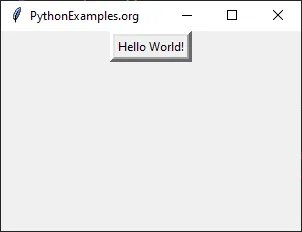
Run on a MacOS.
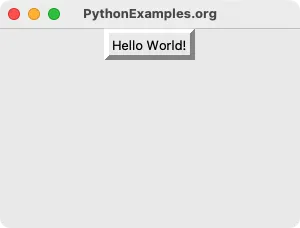
Summary
In this Python Tkinter tutorial, we learned how to set a specific width and height for a Label widget, with examples.