Tkinter Checkbutton – Set value
To programmatically set the value of a Tkinter Checkbutton widget, you can use the set() method of the associated IntVar variable.
For example, consider that we have a Checkbutton checkbutton_1 created with the variable option set to the IntVar checkbutton_1_var.
checkbutton_1 = tk.Checkbutton(window, text="Option 1", variable=checkbutton_1_var)
To set the value of this Checkbutton to 1 (selected), use the set() method as shown below.
checkbutton_1_var.set(1)
Or you can set the value of the Checkbutton to 0 (deselected) as shown below.
checkbutton_1_var.set(0)
In this tutorial, you shall learn how to set the value of a Checkbutton in Tkinter, with examples.
Example
In this example, we shall create three Checkbutton, and a button Set values. When user clicks on the Set values button, we set the first Checkbutton to deselected by setting its value to 0, and set the second and third Checkbuttons to selected by setting their values to 1.
Python Program
import tkinter as tk
def set_values():
checkbutton_1_var.set(0)
checkbutton_2_var.set(1)
checkbutton_3_var.set(1)
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
# Variables for Checkbuttons
checkbutton_1_var = tk.IntVar()
checkbutton_2_var = tk.IntVar()
checkbutton_3_var = tk.IntVar()
# Create Checkbuttons in a loop
checkbutton_1 = tk.Checkbutton(window, text="Option 1", variable=checkbutton_1_var)
checkbutton_2 = tk.Checkbutton(window, text="Option 2", variable=checkbutton_2_var)
checkbutton_3 = tk.Checkbutton(window, text="Option 3", variable=checkbutton_3_var)
checkbutton_1.pack()
checkbutton_2.pack()
checkbutton_3.pack()
button = tk.Button(window, text="Set values", command=set_values)
button.pack()
# Start the Tkinter event loop
window.mainloop()
Output in MacOS
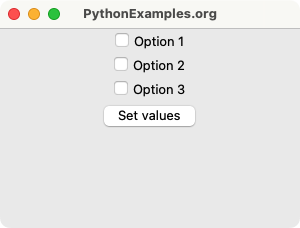
User clicks on the button Set values.
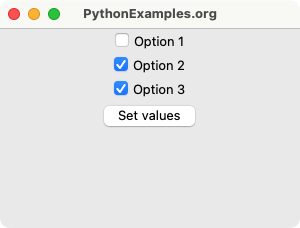
Summary
In this Python Tkinter tutorial, we have seen how to set the values for Checkbutton widgets in Tkinter, with examples.