Tkinter Label – Tooltip
In Tkinter, you can add tooltips to Label widgets to provide additional information or hints when the user hovers the mouse pointer over the label.
To create a tooltip for a Label widget, you can custom implement a Tooltip class that displays a tooltip as shown in the following screenshot.
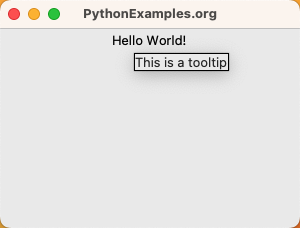
In this tutorial, you will learn how to display a tooltip for a Label widget in Tkinter, with examples.
Tooltip Class
The definition of Tooltip class is given below.
class Tooltip:
def __init__(self, widget, text):
self.widget = widget
self.text = text
self.tooltip = None
self.widget.bind("<Enter>", self.show)
self.widget.bind("<Leave>", self.hide)
def show(self, event=None):
x, y, _, _ = self.widget.bbox("insert")
x += self.widget.winfo_rootx() + 25
y += self.widget.winfo_rooty() + 25
self.tooltip = tk.Toplevel(self.widget)
self.tooltip.wm_overrideredirect(True)
self.tooltip.wm_geometry(f"+{x}+{y}")
label = ttk.Label(self.tooltip, text=self.text, background="#ffffe0", relief="solid", borderwidth=1)
label.pack()
def hide(self, event=None):
if self.tooltip:
self.tooltip.destroy()
self.tooltip = None
Please note that you have to import ttk from tkinter for this class.
This class has a constructor, and two methods. In the constructor we bind the events of mouse entering the label widget area, and mouse leaving the label widget area to the show and hide methods.
The show() method is responsible for creating and positioning the tooltip window relative to the label widget. It creates a new Toplevel window and places a label inside it to display the tooltip text.
The hide() method destroys the tooltip window when the mouse pointer leaves the widget.
Example
In this example, we shall create a Label widget label with some text.
Also, we create an object of type Tooltip with the label widget and tooltip text passed as arguments.
Program
import tkinter as tk
from tkinter import ttk
class Tooltip:
def __init__(self, widget, text):
self.widget = widget
self.text = text
self.tooltip = None
self.widget.bind("<Enter>", self.show)
self.widget.bind("<Leave>", self.hide)
def show(self, event=None):
x, y, _, _ = self.widget.bbox("insert")
x += self.widget.winfo_rootx() + 25
y += self.widget.winfo_rooty() + 25
self.tooltip = tk.Toplevel(self.widget)
self.tooltip.wm_overrideredirect(True)
self.tooltip.wm_geometry(f"+{x}+{y}")
label = ttk.Label(self.tooltip, text=self.text, background="#ffffe0", relief="solid", borderwidth=1)
label.pack()
def hide(self, event=None):
if self.tooltip:
self.tooltip.destroy()
self.tooltip = None
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
# Create a label with tooltip
label = ttk.Label(window, text="Hello World! Hover me.")
label.pack()
# Create a tooltip for the label
tooltip = Tooltip(label, "This is a tooltip")
# Run the application
window.mainloop()
We have set compound="center"
so that the compound of the image and text would be center aligned.
Output
Run on Windows 10.
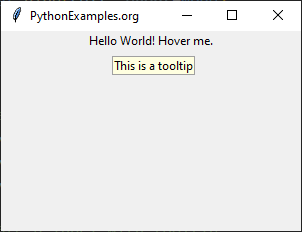
Run on a MacOS.
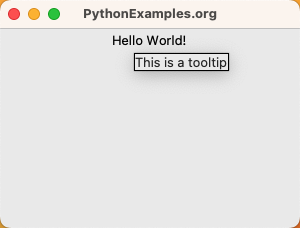
Summary
In this Python Tkinter tutorial, we learned how to display a tooltip for a Label widget, with examples.