⊕
⊖
Contents
Read input from user using Entry
To read input from user using Entry widget in Tkinter, define an Entry widget using Entry() class, and read the text using get() method on the Entry widget.
Code Snippet
The following is a simple skeletal code to define an Entry widget, and read the text from the Entry widget.
tkWindow = Tk()
entry = Entry(tkWindow)
entry.get()
Examples
1. Read name from user
In the following program, we read user name from the user.
We display label, entry, and a button in grid view in the Tkinter window. When user clicks on the button, we call printDetails() method. In printDetails() method, we get the text from the entry widget, and print it to console.
Python Program
from tkinter import *
from functools import partial
def printDetails(usernameEntry) :
usernameText = usernameEntry.get()
print("user entered :", usernameText)
return
# Window
tkWindow = Tk()
tkWindow.geometry('400x150')
tkWindow.title('Python Examples')
# Label
usernameLabel = Label(tkWindow, text="Enter your name")
# Entry for user input
usernameEntry = Entry(tkWindow)
# Define callable function with printDetails function and usernameEntry argument
printDetailsCallable = partial(printDetails, usernameEntry)
# Submit button
submitButton = Button(tkWindow, text="Submit", command=printDetailsCallable)
# Place label, entry, and button in grid
usernameLabel.grid(row=0, column=0)
usernameEntry.grid(row=0, column=1)
submitButton .grid(row=1, column=1)
# Main loop
tkWindow.mainloop()
Output
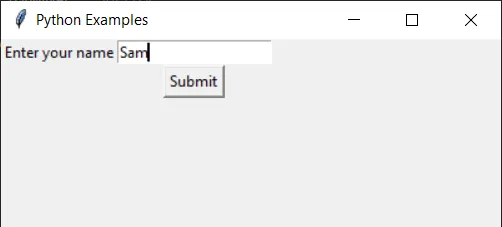
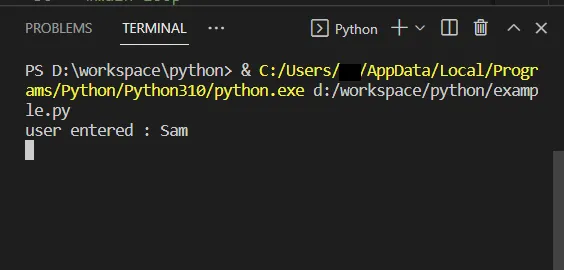
Next Topic ⫸ Tkinter Entry – Password
⫷ Previous Topic Tkinter – Call function on button click