Tkinter Entry – On Enter/Return
In Tkinter, you can handle On Enter/Return key event in an Entry widget.
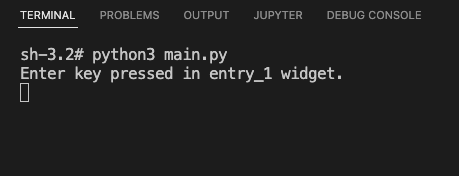
To handle On Enter/Return key event in an Entry widget in Tkinter, bind the Entry widget with Return key and specify the event handler function using Entry.bind() method, as shown below.
entry_1.bind('<Return>', entry_1_return_key_event)
Now, inside the event handler function entry_1_return_key_event, you can write your code on how to handle this event, or what to happen on this event. In this tutorial, we shall just print a statement that the event has happened.
def entry_1_return_key_event(event):
print('Enter key pressed in entry_1 widget.')
In this tutorial, you will learn how to set an event handler function for On Enter/Return key event in Entry widget, with examples.
Examples
1. Set event handler for Return key event in Entry widget
In this example, we create a basic Tk window with an Entry widget entry_1. We bind the <Return> key event to the Entry widget. If the user clicks Enter or Return key while entering a value in first Entry widget, the event is triggered and executes entry_1_return_key_event function.
Python Program
import tkinter as tk
def entry_1_return_key_event(event):
print('Enter key pressed in entry_1 widget.')
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
label = tk.Label(window, text="Enter input")
label.pack()
# First Entry widget
entry_1 = tk.Entry(window)
entry_1.bind('<Return>', entry_1_return_key_event)
entry_1.pack()
# Run the application
window.mainloop()
Output in Windows
Output in MacOS
Run the program.
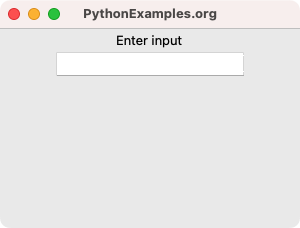
Enter some value, and press Enter/Return key.
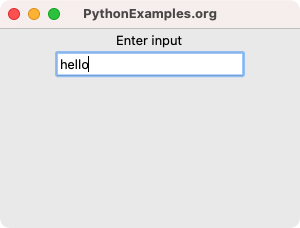
The event handler function entry_1_return_key_event executes.
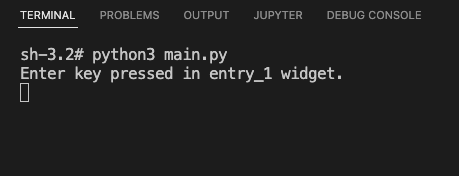
Summary
In this Python Tkinter tutorial, we have seen how to handle on Enter/Return key event in Entry widget in Tkinter, with examples.