Tkinter Checkbutton – On change event
To execute a function whenever there is a change in the value of the Checkbutton in Tkinter, or in other words whenever the Checkbutton is selected or unselected, set the command option of the Checkbutton with the required callback function.
Checkbutton(window, command=on_checkbutton_toggle, ...)
Whenever this Checkbutton is selected or unselected, given on_checkbutton_toggle function is called. And inside this function, we can get the status of selection by checking the variable associated with this Checkbutton.
In this tutorial, you shall learn how to set a command function to execute when the selection status of the Checkbutton is changed, with examples.
Example
In the following example, we shall create a Checkbutton widget, and assign a command function on_checkbutton_toggle.
Python Program
import tkinter as tk
def on_checkbutton_toggle():
if checkbutton_var.get():
print("Checkbutton is selected")
else:
print("Checkbutton is deselected")
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
# Create a variable to store the checkbutton state
checkbutton_var = tk.IntVar()
# Create the checkbutton widget
checkbutton_1 = tk.Checkbutton(window, text="Option 1", variable=checkbutton_var, command=on_checkbutton_toggle)
# Pack the checkbutton widget
checkbutton_1.pack()
# Start the Tkinter event loop
window.mainloop()
Output in Windows
GUI when then checkbutton is checked.
Output in Mac
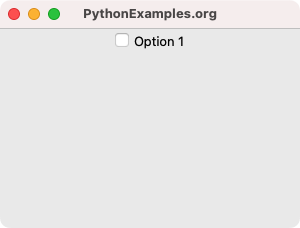
Now, check the checkbutton, by clicking on it using mouse.
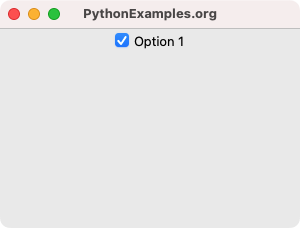
The state of the checkbutton changes, and the callback function on_checkbutton_toggle is called. Since the checkbutton_1 is checked, checkbutton_var.get() returns value of 1, and the if-block is run.
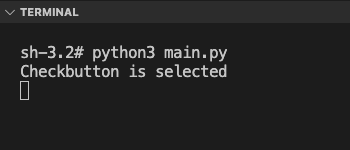
Now, deselect the Checkbutton, by clicking on the checked box.
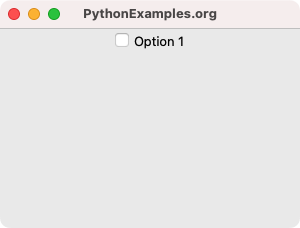
The state of the checkbutton changes, and the callback function on_checkbutton_toggle is called. Since the checkbutton_1 is unchecked, checkbutton_var.get() returns value of 0, and the else-block is run.
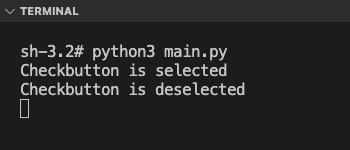
Summary
In this Python Tkinter tutorial, we have seen how to handle the event of selection and deselection of a Checkbutton widget, with examples.