Change Tkinter Button Background Color - Python Examples
Python Tkinter Button Background Color
You can change the background color of a Tkinter Button by setting the bg
property of Tkinter Button with a color string or HEX value.
Assign any standard color or rgb hex value to the bg
property as shown below.
button = Button(tkWindow, bg='blue')
button = Button(tkWindow, bg='black')
button = Button(tkWindow, bg='white')
button = Button(tkWindow, bg='red')
#hex values
button = Button(tkWindow, bg='#54FA9B')
button = Button(tkWindow, bg='#A877BA')
The default color of Tkinter Button is grey. In this tutorial, we will learn how to change the color of Tkinter Button.
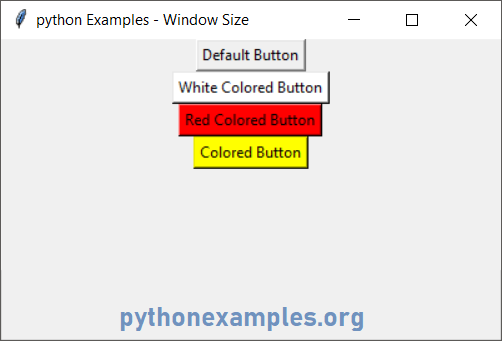
Examples
1. Set Tkinter Button color to blue
In this example, we have set the Button's background color to blue by assigning named property bg
in Button()
class to blue
.
Python Program
from tkinter import *
tkWindow = Tk()
tkWindow.geometry('400x150')
tkWindow.title('Button Background Example')
button = Button(tkWindow, text = 'Submit', bg='blue', fg='white')
button.pack()
tkWindow.mainloop()
Output
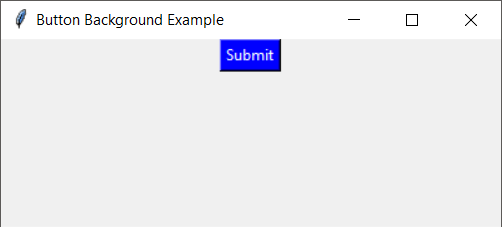
2. Set Tkinter Button background color to black
In this example, we have set the background color of the button to black with bg='black'
.
Python Program
from tkinter import *
tkWindow = Tk()
tkWindow.geometry('400x150')
tkWindow.title('Button Background Example')
button = Button(tkWindow, text = 'Submit', bg='black', fg='white')
button.pack()
tkWindow.mainloop()
Output
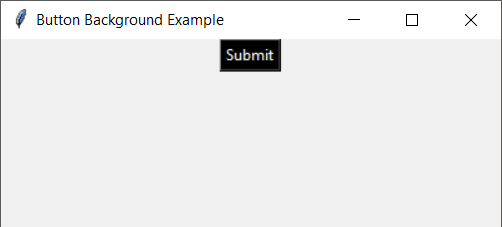
3. Set Tkinter Button background color to Hex color
You can also assign any RGB equivalent hex color to the bg
property.
In this example, we assigned bg='#ffb3fe'
.
Python Program
from tkinter import *
tkWindow = Tk()
tkWindow.geometry('400x150')
tkWindow.title('Button Background Example')
button = Button(tkWindow, text = 'Submit', bg='#ffb3fe')
button.pack()
tkWindow.mainloop()
Output
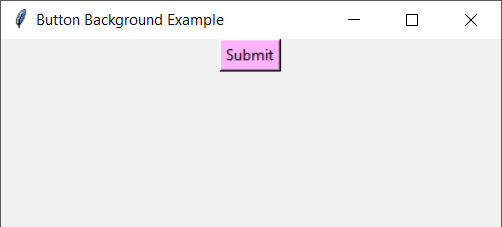
Summary
In this tutorial of Python Examples, we learned to set the background color of Tkinter button.