Contents
Tkinter Canvas – Create Rectangle
In Tkinter, you can create a rectangle on Canvas widget with top left corner at specific (x1, y1) coordinate and bottom right corner at specific (x2, y2) coordinate.
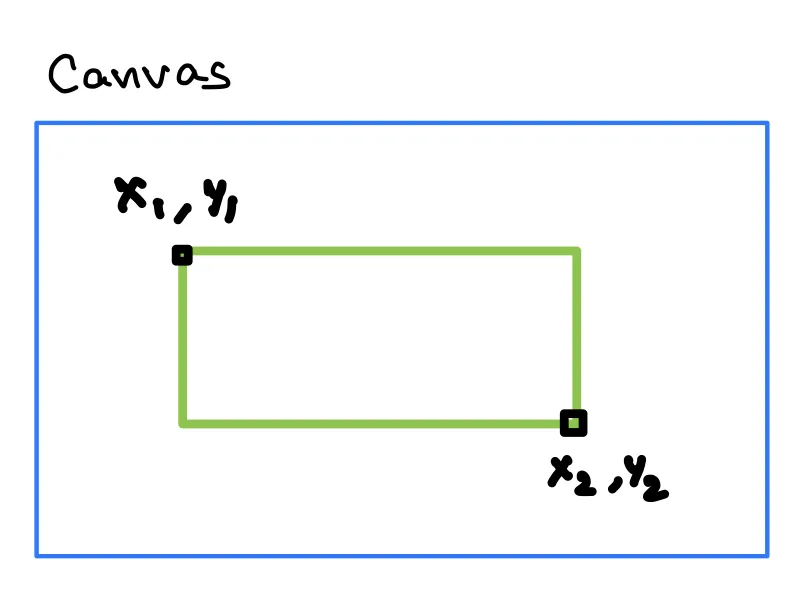
To create a rectangle in a Canvas widget in Tkinter, you can use the create_rectangle() method of the Canvas class.
create_rectangle() method takes the coordinates of two points as arguments and returns an identifier for the created rectangle object on the canvas. The first point (x1, y1) defines the top left corner coordinates of the rectangle and the second point (x2, y2) defines the bottom right corner coordinates of the rectangle, as shown in the above diagram.
To create or draw a rectangle from (x1, y1) coordinate to (x2, y2) coordinate on the Canvas, use the following syntax.
Canvas.create_rectangle(x1, y1, x2, y2)
We can also specify other options to the method like fill color, width of the line, etc.
In this tutorial, you shall learn how to create a rectangle on the Canvas widget in Tkinter, with examples.
Examples
1. Create rectangle from (50, 50) to (200, 150) on Canvas
In this example, we shall create a Canvas canvas_1, and create a line on this Canvas from (10, 10) to (200,150).
Python Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
# Create a Canvas widget
canvas_1 = tk.Canvas(window, width=300, height=200)
canvas_1.pack()
# Create a line on the canvas
rectangle_1 = canvas_1.create_rectangle(50, 50, 200, 150)
window.mainloop()
CopyOutput in MacOS
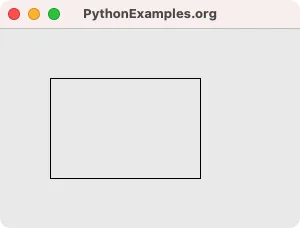
2. Create rectangle on Canvas, with specific fill color
In this example, we shall create a line on Canvas, with a fill color of red, and width of 10.
Python Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
# Create a Canvas widget
canvas_1 = tk.Canvas(window, width=300, height=200)
canvas_1.pack()
# Create a line on the canvas
rectangle_1 = canvas_1.create_rectangle(50, 50, 200, 150, fill = "lightgreen")
window.mainloop()
CopyOutput in MacOS
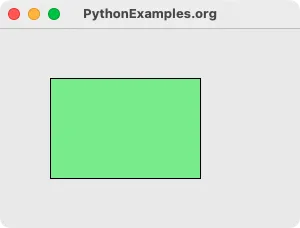
Summary
In this Python Tkinter tutorial, we have seen how to create a rectangle on Canvas widget in Tkinter, with examples.