Tkinter – Context Menu
In Tkinter, context menus or popup menus are the menus that appear when user right-clicks on a widget or specific area of an application.
To create a context menu in Tkinter, you can use Tk.Menu class. To bind the context menu to a widget, you can use bind() method.
The following is a simple code snippet of how we create a context menu and bind it to a label. This is not complete code, but gives you an idea of how to create a context menu, and bind it to a label widget for a right click mouse event.
def show_context_menu(event):
context_menu.post(event.x_root, event.y_root)
context_menu = tk.Menu(window, tearoff=0)
label.bind("<Button-3>", show_context_menu)
When user right-clicks on the label, the context menu is displayed.
Steps to create Context Menu in Tkinter
In this tutorial, we will consider the following use case.
There is a label widget. When user clicks on this label widget, a context menu appears with a single item in the menu. When user the menu item, a message is displayed in the console output.
Step 1
Create Label widget.
label = tk.Label(window, text="Right-click me!")
Step 2
Create context menu using Tk.Menu class.
context_menu = tk.Menu(window, tearoff=0)
Step 3
Add items to the context menu, and specify the command function.
context_menu.add_command(label="Action", command=menu_action)
The command function menu_action() should also be defined prior to the specifying it as callback function to the menu item.
def menu_action():
print("Context menu action.")
Step 4
Bind the context menu to the label widget. To bind our context menu to the label, you can use bind() method as shown below.
label.bind("<Button-3>", show_context_menu)
The first argument is the sequence “<Button-3>” which specifies right click as the trigger for executing the function specified in the second argument show_context_menu.
The function show_context_menu handles the right click event. And we would like to the show the context_menu where the click happened. Therefore, the function show_context_menu goes like this.
def show_context_menu(event):
context_menu.post(event.x_root, event.y_root)
Example
The following is the complete program by combining all the above steps to create and display a context menu when user right clicks on the label.
Python Program
import tkinter as tk
def show_context_menu(event):
context_menu.post(event.x_root, event.y_root)
def menu_action():
print("Context menu action")
# Create the main window
window = tk.Tk()
# Create a label widget
label = tk.Label(window, text="Right-click me!")
label.pack()
# Create the context menu
context_menu = tk.Menu(window, tearoff=0)
context_menu.add_command(label="Action", command=menu_action)
# Bind the context menu to the label widget
label.bind("<Button-3>", show_context_menu)
# Run the application
window.mainloop()
Output
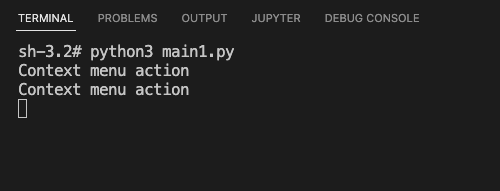
Summary
In this Python Tkinter tutorial, we learned how to create a Context menu or Popup menu in Tkinter, with the help of examples.