Create Menu from a List in Tkinter
In Tkinter, you can create menu a complete menu for your application from a list of lists by iterating over the lists, and adding each of the item in the list as a menu item in the menu.
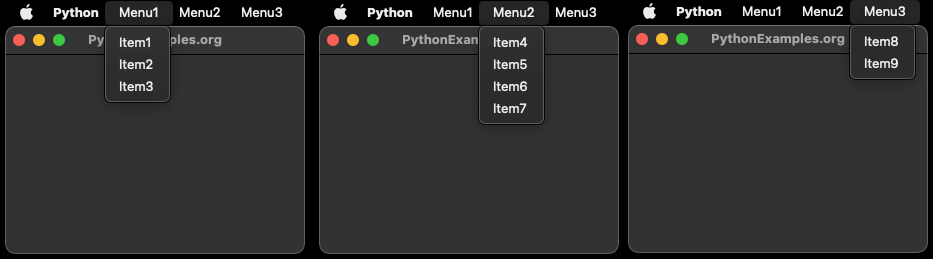
As an input we get
- A list containing the labels for menus in the menu bar.
- A list of lists for the items in each menu.
In this tutorial, you will learn how to create a whole menu from a list of lists.
Steps to create a whole Menu from a List of Lists in Tkinter
Step 1
Take a list of strings that can be used for labels of menus in menu bar.
menus_list = ['Menu1', 'Menu2', 'Menu3']
Take a list of lists that can be used for menu items in menus.
menu_items_list = [
['Item1', 'Item2', 'Item3'],
['Item4', 'Item5', 'Item6', 'Item7'],
['Item8', 'Item9']
]
Step 2
Zip both menus_list and menu_items_list, and iterate over the the items. For each iteration, we create a menu in the menu bar.
# Iterate over all menus
for (menu_label, items) in zip(menus_list, menu_items_list):
# Create menu
menu = tk.Menu(menu_bar, tearoff=0)
for item in items:
menu.add_command(label=item, command=lambda x=item:menu_item_action(x))
# Add the menu to the menu bar
menu_bar.add_cascade(label=menu_label, menu=menu)
The inner For loop adds the menu items to the menu.
Example
In this example, we shall create the whole menu in the menu bar from a list of menus in the menu bar, and list of lists for the menu items.
We have specified a single callback function for all the menu items, that takes the label of the menu item as an input. If you want, you can specify another list of lists with the callback functions, zip it along with the menu labels, and menu item labels, and specify the command for each menu item in the For loop.
Program
import tkinter as tk
def menu_item_action(arg):
print(f'You clicked {arg}.')
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
# Create the menu bar
menu_bar = tk.Menu(window)
# Input lists for creating menus
menus_list = ['Menu1', 'Menu2', 'Menu3']
menu_items_list = [
['Item1', 'Item2', 'Item3'],
['Item4', 'Item5', 'Item6', 'Item7'],
['Item8', 'Item9']
]
# Iterate over all menus
for (menu_label, items) in zip(menus_list, menu_items_list):
# Create menu
menu = tk.Menu(menu_bar, tearoff=0)
for item in items:
menu.add_command(label=item, command=lambda x=item:menu_item_action(x))
# Add the menu to the menu bar
menu_bar.add_cascade(label=menu_label, menu=menu)
# Attach the menu bar to the main window
window.config(menu=menu_bar)
# Start the Tkinter event loop
window.mainloop()
Output
Run on a MacOS.
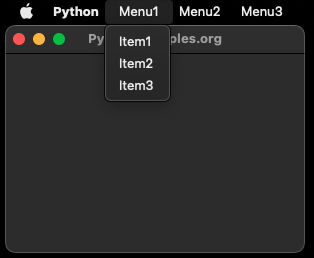
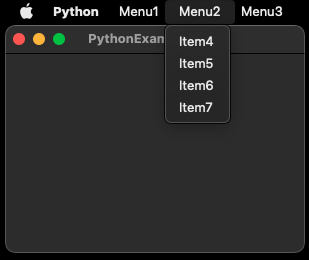
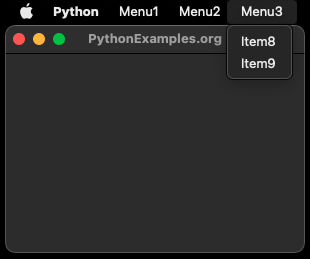
Summary
In this Python Tkinter tutorial, we learned how to create a complete menu from a list of lists, with examples.