Create Menu from a List in Tkinter
In Tkinter, you can create menu items of a menu from a list by iterating over the given list, and adding each of the item in the list as a menu item in the menu.
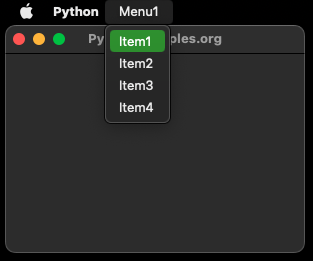
In this tutorial, you will learn how to create a menu where the menu items are taken from a list, with examples.
Steps to create a Menu from a List in Tkinter
Step 1
Define a function that can be used for the callback function for the menu items.
def menu_item_action(arg):
print(f'You clicked {arg}.')
Inside the callback function, we print the menu item on which the user clicked.
Step 2
Create a menu menu_1 in menu bar using Tk.Menu class.
menu_bar = tk.Menu(window)
menu_1 = tk.Menu(menu_bar, tearoff=False)
Step 3
Take a list of strings, that are considered for labels of menu items.
my_list = ['Item1', 'Item2', 'Item3', 'Item4']
Step 4
Use a Python For loop to iterate over the given list, and add a menu item for each of the item in the list.
for item_label in my_list:
menu_1.add_command(label=item_label, command=lambda x=item_label:menu_item_action(x))
Step 5
Cascade menu menu_1 to the menu bar, and configure the Tk window with the menu bar.
Example
In this example, we shall create a menu menu_1 in the menu bar and populate the menu items from the elements of a list my_list.
When user clicks on a menu item, the callback function is called, and we print the label of the menu item, which the user clicked.
Program
import tkinter as tk
def menu_item_action(arg):
print(f'You clicked {arg}.')
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
# Create the menu bar
menu_bar = tk.Menu(window)
# Create the menu
menu_1 = tk.Menu(menu_bar, tearoff=0)
my_list = ['Item1', 'Item2', 'Item3', 'Item4']
# Add items for Menu1
for item_label in my_list:
menu_1.add_command(label=item_label, command=lambda x=item_label:menu_item_action(x))
# Add the menu to the menu bar
menu_bar.add_cascade(label="Menu1", menu=menu_1)
# Attach the menu bar to the main window
window.config(menu=menu_bar)
# Start the Tkinter event loop
window.mainloop()
Output
Run on a MacOS.
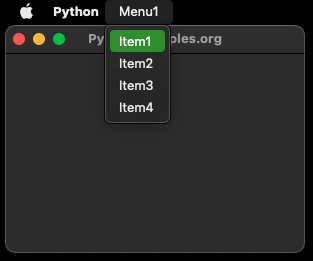
Click on an item.
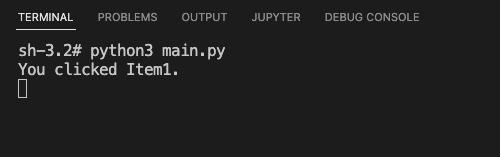
Summary
In this Python Tkinter tutorial, we learned how to create a menu from a list, with examples.