Contents
Tkinter filedialog – Ask user to open a file
In Tkinter, the filedialog.askopenfile() method is used to display a file dialog to open a file, and return the file object.
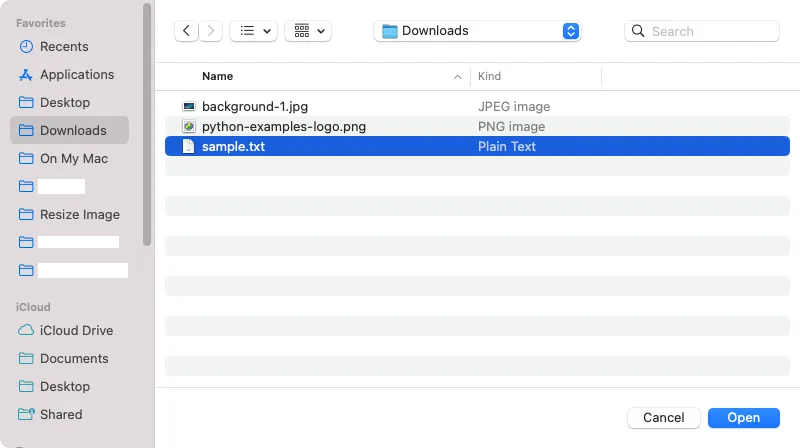
In this tutorial, you will learn how to display a file dialog asking the user to open a file, using filedialog.askopenfile() method in Tkinter, with an example program.
A sample code snippet to use askopenfile() method is
from tkinter.filedialog import askopenfile
filedialog.askopenfile()
No need to pass any arguments. But there are optional parameters that you can pass, to modify the behavior of the file dialog. We shall go through some of the generally used optional parameters in our next tutorials.
Examples
1. Select a text file in file dialog, and print its contents
Consider a simple use case where you would like to get the name of the user by prompting for input, read the input, and print a personalized greeting on the main window.
Python Program
import tkinter as tk
from tkinter.filedialog import askopenfile
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
def open_file():
file = askopenfile()
if file:
lines = file.readlines()
print(lines)
else:
print("No file selected.")
# Create a button
button = tk.Button(window, text="Open File", command=open_file)
button.pack()
# Run the application
window.mainloop()
Output in Windows
Output in MacOS
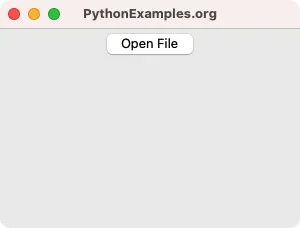
Click on the Open File button. A file dialog appears as shown in the following.
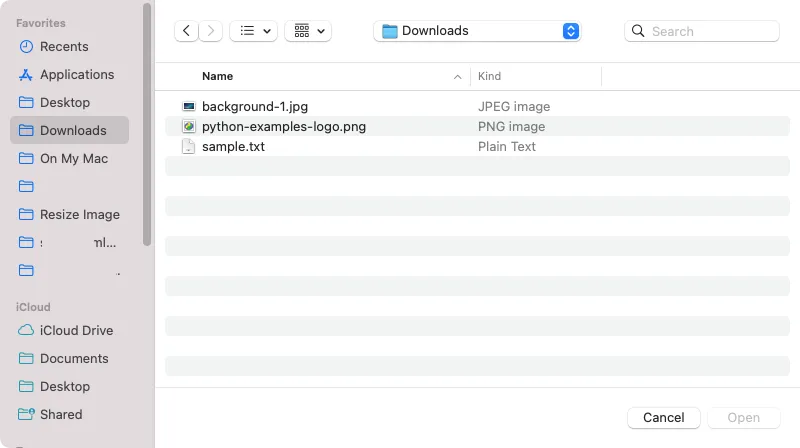
Browse, and select a file. For this example, we select a Plain Text file.
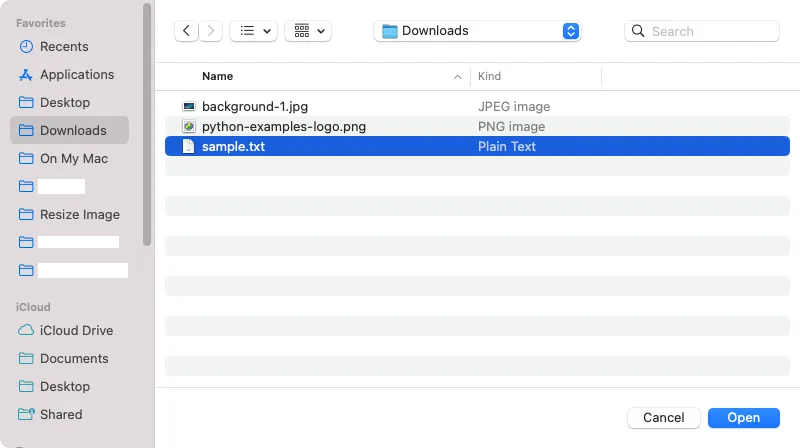
And click on the Open button. The askopenfile() returns the file object. We read the lines in the file object, and print it to standard output.
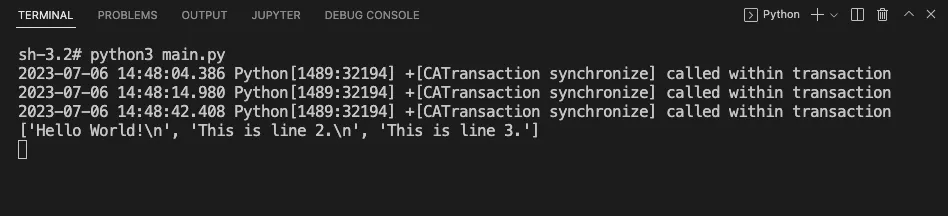
If user clicks on Cancel button in the file dialog, then askopenfile() returns None, and the else block in our open_file() function would be executed as shown in the following Terminal output.
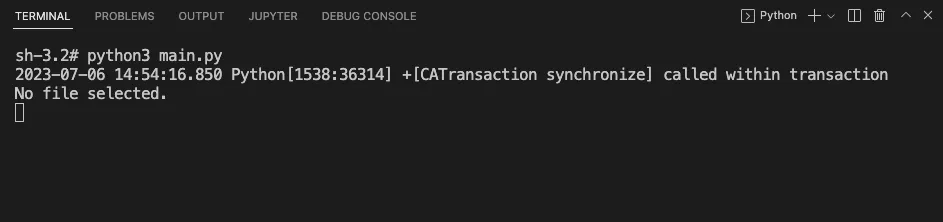
Summary
In this Python Tkinter tutorial, we learned how to display a file dialog to open a file in Tkinter, with examples.