Tkinter Frame widget
In this tutorial, you shall learn about Frame container widget in TKinter: What is a Frame widget, how to create a Frame widget, and an example for Frame widget.
What is Frame widget in Tkinter
Frame is a container widget that acts as a rectangular region to hold other widgets or groups of widgets. It provides a way to organize and group multiple widgets together.
The Frame widget does not have any visible appearance of its own but serves as a container for other widgets.
The following is a screenshot of the Frame widget with a specific background color, and a label widget inside this Frame widget.
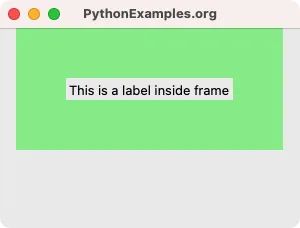
How to create a Frame widget in Tkinter?
To create a Frame widget in Tkinter, you can use the Frame class constructor. The parent window must be passed as the first argument, and this is the mandatory parameter. Rest of the parameters are optional and can be passed as keyword arguments, or you can set these options after initializing the Frame widget object.
A simple example to create a Frame widget is given below.
import tkinter as tk
window = tk.Tk()
frame_1 = tk.Frame(window)
You can also pass keyword arguments like width, height, background, etc..
frame_1 = tk.Frame(window, background='lightgreen', width=250, height=150)
Copy- window specifies the parent for this Frame widget.
- width specifies the number of pixels along the horizontal axis.
- height specifies the number of pixels along the vertical axis.
Example for Frame widget in Tkinter
In this example, we shall create a simple GUI application using Tkinter with a Frame widget, and create a Label widget inside this Frame widget.
By default, a Frame shrinks to its contents. To visualize the Frame widget in the window, we set its padding to 50 pixels, and set the background color to lightgreen.
Python Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
# Create a Frame widget
frame_1 = tk.Frame(window, background='lightgreen', padx=50, pady=50)
frame_1.pack()
# Create some widgets inside the frame
label_1 = tk.Label(frame_1, text='This is a label inside frame')
label_1.pack()
window.mainloop()
Output in MacOS
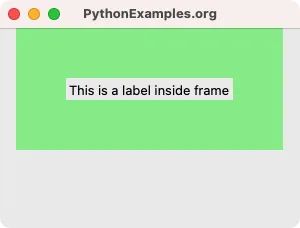
Summary
In this Python Tkinter tutorial, we have seen what a Frame widget is, how to create a Frame widget, and an example for Frame widget with a label widget in it.