Tkinter Listbox widget
In this tutorial, you shall learn about Listbox widget in TKinter: What is a Listbox widget, how to create a Listbox widget, and an example for Listbox widget.
What is Listbox widget in Tkinter
Listbox widget is used to display a list of items from which the user can select one or more. It provides a scrollable list of items that can be clicked or selected using keyboard navigation.
In a GUI, a Listbox widget with default parameters/options would be as shown in the following screenshot.
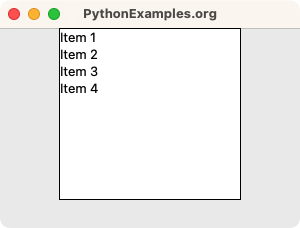
By default, user can select an item by clicking on the item. You can also set the Listbox widget to select multiple items using selectmode option. There are other options as well that affect the behavior or styling of the Listbox widget. We shall go through them in the following tutorials.
How to create a Listbox widget in Tkinter?
To create a Listbox widget in Tkinter, you can use the Listbox class constructor. The parent window must be passed as the first argument, and this is the mandatory parameter. Rest of the parameters are optional and can be passed as keyword arguments, or you can set them after initializing the Listbox widget object.
A simple example to create a Listbox widget is given below.
import tkinter as tk
window = tk.Tk()
listbox_1 = tk.Listbox(window)
Now, let us specify some of the parameters.
listbox = tk.Listbox(window, height=3, selectmode=tk.MULTIPLE)
- window specifies the parent for this Scale widget.
- height specifies the number of lines in the Listbox. If the number of items is more than the specified height, you can scroll through the items.
- selectmode can be set to
tk.SINGLE
(default) for single item selection ortk.MULTIPLE
for multiple item selection.
Example for Listbox widget in Tkinter
In this example, we shall create a simple GUI application using Tkinter with a Listbox widget.
Also, we have bind the event of selecting the items in the Listbox to a callback function on_selection.
When an item is selected, the selected item is printed to the console.
Python Program
import tkinter as tk
def on_selection(event):
selected_indices = listbox.curselection()
for index in selected_indices:
print("Selected item:", listbox.get(index))
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
listbox = tk.Listbox(window)
listbox.pack()
# Inserting items into the listbox
listbox.insert(tk.END, "Item 1")
listbox.insert(tk.END, "Item 2")
listbox.insert(tk.END, "Item 3")
listbox.insert(tk.END, "Item 4")
# Binding the selection event
listbox.bind("<<ListboxSelect>>", on_selection)
window.mainloop()
Output in MacOS
Run the program.
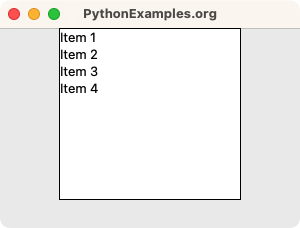
User selects the Item 2.
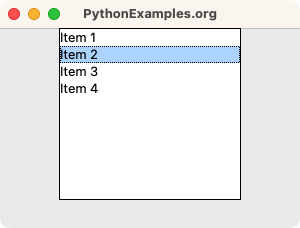
The callback function is called, and prints the selected item to standard output.
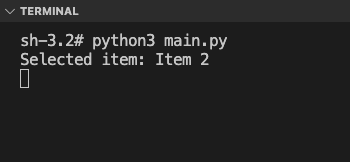
Summary
In this Python Tkinter tutorial, we have seen what a Listbox widget is, how to create a Listbox widget, and an example for Listbox widget.