Tkinter Message Box – Without buttons
In Tkinter, the messagebox is a modal dialog with standardised buttons.
But, we can create a custom message box without any buttons as shown in the following screenshot.
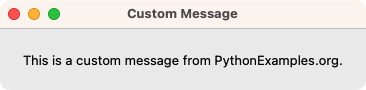
To display a message box without any buttons, it is recommended to create a top level widget with the present window.
dialog = tk.Toplevel(window)
Then we can set its title and display a message in this using a Label.
dialog.title(title)
label = tk.Label(dialog, text=message)
label.pack(padx=20, pady=20)
And then we instruct the window manager that this widget is transient with regard to our main window.
dialog.transient()
This way, the dialog stays on top of our main window, hides with it, doesn’t show up on the taskbar, etc.
Also, we shall bind this dialog to Escape key stroke, so that it is gone when user clicks Escape key from keyboard.
In this tutorial, you will learn how to display a custom message box with no buttons, with example.
Examples
1. Print the return value of showinfo() method
In this example, we define a function show_custom_message() to display a custom message box with given title and message.
Python Program
import tkinter as tk
# Create the main window
window = tk.Tk()
# Define a function to create a custom message box
def show_custom_message(title, message):
# Create a top-level window
dialog = tk.Toplevel(window)
dialog.title(title)
dialog.transient()
# Create a label with the message
label = tk.Label(dialog, text=message)
label.pack(padx=20, pady=20)
# This window closes when user hits Escape key
dialog.bind('<Escape>', lambda e, w=dialog: w.destroy())
# Run the dialog window
dialog.mainloop()
# Call the function to display a custom message box
show_custom_message("Custom Message", "This is a custom message from PythonExamples.org.")
# Run the application
window.mainloop()
Output
In Windows
In MacOS
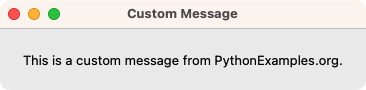
If user presses Escape key, then this dialog or message box or whatever is gone.
Summary
In this Python Tkinter tutorial, we learned how to display a message box without buttons in Tkinter, with examples.