Tkinter messagebox – Ask Yes, No, or Cancel
In Tkinter, the messagebox.askyesnocancel() method is used to ask the user a question with three actions: Yes, No, and Cancel.
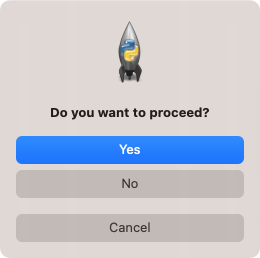
If user clicks on Yes button, then askyesnocancel() returns True, or else if user clicks on No button, then askyesnocancel() returns False, or else if user clicks on Cancel button, then askyesnocancel() returns None. Based on the returned value, we can proceed with the required logic.
In this tutorial, you will learn how to ask a question in a message box using messagebox.askyesnocancel() method in Tkinter, with an example program.
Syntax of messagebox.askyesnocancel()
The syntax of askyesnocancel() method is
messagebox.askyesnocancel(title, message)
where
Parameter | Description |
---|---|
title | This string is displayed as title of the messagebox. [How a title is displayed in message box depends on the Operating System. ] |
message | This string is displayed as a message inside the message box. |
Examples
1. Ask user a question using messagebox
For example, consider that you have created a GUI application using Python Tkinter, and you would like to ask the user a question: Do you want to proceed?
And you would like to ask the question with three possible actions from user: Yes, No, or Cancel.
Python Program
import tkinter as tk
from tkinter import messagebox
# Create the main window
window = tk.Tk()
# Ask a question: Yes, No, or Cancel
result = messagebox.askyesnocancel("Confirmation", "Do you want to proceed?")
print(result)
if result is True:
print("User clicked Yes")
elif result is False:
print("User clicked No")
elif result is None:
print("User clicked Cancel")
# Run the application
window.mainloop()
After user takes an action by clicking on any of the buttons, the return value is stored in result variable. Based on this value, we print an output.
Output
In Windows
In MacOS
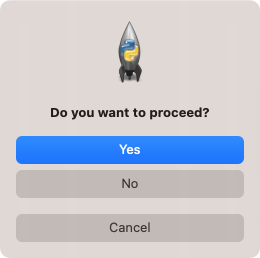
If user clicks on Yes button, then we get the following output in Terminal.
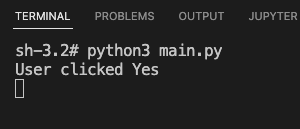
If user clicks on No button, then we get the following output in Terminal.
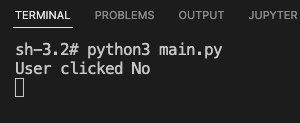
If user clicks on Cancel button, then we get the following output in Terminal.
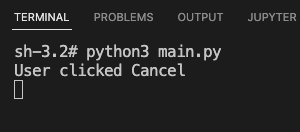
Summary
In this Python Tkinter tutorial, we learned how to ask a question with Yes, No, and Cancel actions in a message box in Tkinter, with examples.