Tkinter messagebox - Ask Yes or No
Tkinter messagebox – Ask Yes or No
In Tkinter, the messagebox.askyesno() method is used to ask the user a question with two buttons: Yes, No.
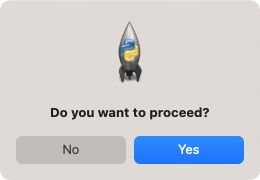
If user clicks on Yes button, then askyesno() returns True, or else if user clicks on No button, then askyesno() returns False. Based on the returned value, we can proceed with the required logic.
In this tutorial, you will learn how to ask a question in a message box using messagebox.askyesno() method in Tkinter, with an example program.
Syntax of messagebox.askyesno()
The syntax of askyesno() method is
messagebox.askyesno(title, message)
where
Parameter | Description |
---|---|
title | This string is displayed as title of the messagebox. [How a title is displayed in message box depends on the Operating System. ] |
message | This string is displayed as a message inside the message box. |
Examples
1. Ask if user want to proceed with the operation
For example, consider that you have created a GUI application using Python Tkinter, and you would like to ask the user a question: Do you want to proceed?
You can ask the question with Yes or No actions in a messagebox, as shown in the following code.
Python Program
import tkinter as tk
from tkinter import messagebox
# Create the main window
window = tk.Tk()
# Ask for Yes or No confirmation
result = messagebox.askyesno("Confirmation", "Do you want to proceed?")
if result:
print("User clicked Yes")
else:
print("User clicked No")
# Run the application
window.mainloop()
After user takes an action by clicking on either of the buttons, the return value is stored in result variable. Based on this value, we print an output.
Output
In Windows
In MacOS
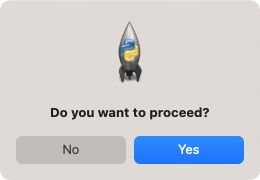
If user clicks on Yes, then we get the following output in Terminal.
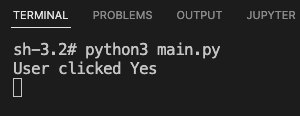
If user clicks on No, then we get the following output in Terminal.
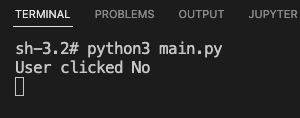
Summary
In this Python Tkinter tutorial, we learned how to ask a question with Yes and No actions in a message box in Tkinter, with examples.