Tkinter Radiobutton – Check if an option is selected
When we create a group of Radiobutton widgets, we set a specific variable to all the radio buttons in the group. If a radio button is selected, we get the value of the selected radio button from this variable. If no radio button is selected, then we get an empty string from this variable.
To check if a radio button is selected or not from a Radiobutton group in Tkinter, check if the value in the variable is an empty string or not.
In this tutorial, you will learn how to check if a Radiobutton is selected or not in a Radiobutton group in Tkinter, with example program.
Example
In this example, we shall create a group of three Radiobutton widgets in a Tk window. We have also a button Check if an Option is Selected. When user clicks on this button, we get the value from the Radiobutton group variable radio_var, and check if this value is an empty string or not in an if else statement.
Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
# Create a variable to hold the selected option
radio_var = tk.StringVar()
# Create Radiobuttons
radio_button1 = tk.Radiobutton(window, text="Option 1", variable=radio_var, value="Option 1")
radio_button2 = tk.Radiobutton(window, text="Option 2", variable=radio_var, value="Option 2")
radio_button3 = tk.Radiobutton(window, text="Option 3", variable=radio_var, value="Option 3")
# Pack the Radiobuttons
radio_button1.pack()
radio_button2.pack()
radio_button3.pack()
def check_if_option_selected():
selected_option = radio_var.get()
if selected_option == "":
print("No option selected.")
else:
print(f"Selected option : {selected_option}")
# Button widget
clear_button = tk.Button(window, text="Check if an Option is Selected", command=check_if_option_selected)
clear_button.pack()
# Start the Tkinter event loop
window.mainloop()
Output in Windows
Output on MacOS
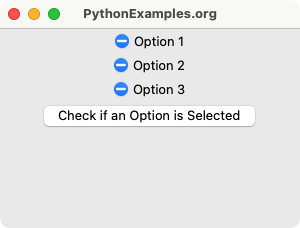
User clicks on Check if an Option is Selected button.
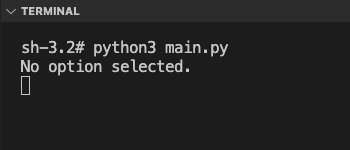
User selects “Option 2”.
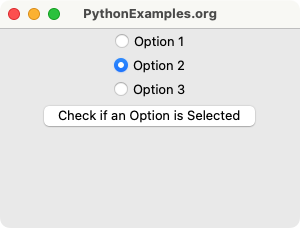
User clicks on Check if an Option is Selected button.
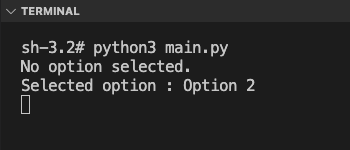
Summary
In this Python Tkinter tutorial, we learned how to check if a Radiobutton is selected or not in a group in Tkinter, with examples.