Tkinter Radiobutton – Clear Selection
To clear the selection of Radiobutton widgets in Tkinter, set the variable for the Radiobutton group with an empty string. This will deselect all the radio buttons within the group.
In this tutorial, you will learn how to clear the selection of radio buttons in a groups of Radiobutton widgets, in a Tk window, with example program.
Example
In this example, we shall create a group of three Radiobutton widgets in a Tk window, and a Button widget Clear Selection. When user clicks on the button Clear Selection, the selected Radiobutton option is cleared, and the initial unselected state is set.
Program
import tkinter as tk
def on_select():
selected_option = radio_var.get()
print(f"Selected option: {selected_option}")
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
# Create a variable to hold the selected option
radio_var = tk.StringVar()
# Create Radiobuttons
radio_button1 = tk.Radiobutton(window, text="Option 1", variable=radio_var, value="Option 1", command=on_select)
radio_button2 = tk.Radiobutton(window, text="Option 2", variable=radio_var, value="Option 2", command=on_select)
radio_button3 = tk.Radiobutton(window, text="Option 3", variable=radio_var, value="Option 3", command=on_select)
# Pack the Radiobuttons
radio_button1.pack()
radio_button2.pack()
radio_button3.pack()
def clear_selection():
radio_var.set("")
print("Selection cleared")
# Clear selection button
clear_button = tk.Button(window, text="Clear Selection", command=clear_selection)
clear_button.pack()
# Start the Tkinter event loop
window.mainloop()
Output in Windows
Output on MacOS
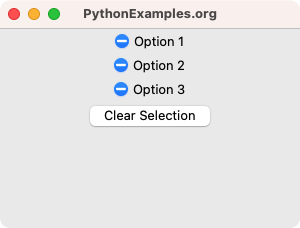
User selects “Option 2” from.
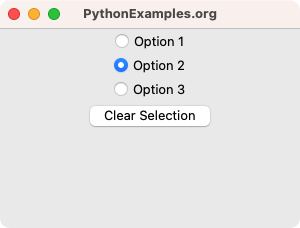
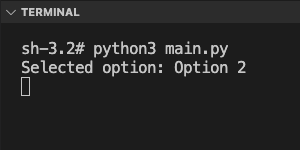
User clicks on the the Clear Selection button.
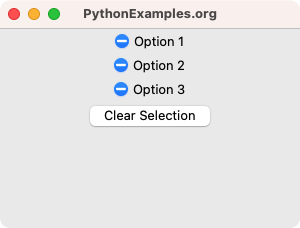
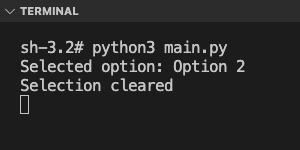
Summary
In this Python Tkinter tutorial, we learned how to clear the selection of Radiobutton widgets in a group, with examples.