Tkinter Radiobutton – Get selected value
When we create a group of Radiobutton widgets, we set a specific variable to all the radio buttons in the group. We can get the selected value from this variable.
To get the selected value of a Radiobutton widgets group in Tkinter, call get() method on the variable used for all the buttons in the Radiobutton group. This method returns the value of the selected Radiobutton.
In this tutorial, you will learn how to get the value of selected radio button in a group in Tkinter, with example program.
Example
In this example, we shall create a group of three Radiobutton widgets in a Tk window. We have also a button Get Selected Option. When user clicks on this button, we get the value of selected Radiobutton, and print this value to the standard output.
Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
# Create a variable to hold the selected option
radio_var = tk.StringVar()
# Create Radiobuttons
radio_button1 = tk.Radiobutton(window, text="Option 1", variable=radio_var, value="Option 1")
radio_button2 = tk.Radiobutton(window, text="Option 2", variable=radio_var, value="Option 2")
radio_button3 = tk.Radiobutton(window, text="Option 3", variable=radio_var, value="Option 3")
# Pack the Radiobuttons
radio_button1.pack()
radio_button2.pack()
radio_button3.pack()
def get_selected_option():
selected_option = radio_var.get()
print(f"Selected option : {selected_option}")
# Button widget
clear_button = tk.Button(window, text="Get Selected Option", command=get_selected_option)
clear_button.pack()
# Start the Tkinter event loop
window.mainloop()
Output in Windows
Output on MacOS
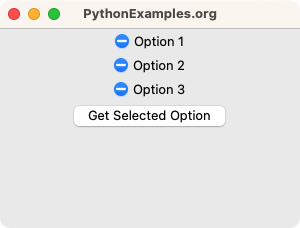
User selects “Option 2”.
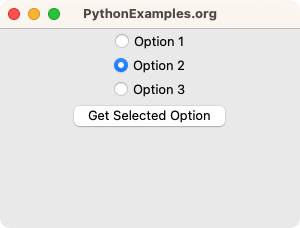
User clicks on “Get Selected Option” button.
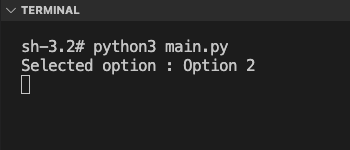
Summary
In this Python Tkinter tutorial, we learned how to get the value of selected Radiobutton in a group, with examples.