Tkinter Radiobutton Groups
Tkinter Radiobutton Groups
To create different groups of Radiobutton widgets in Tkinter, give a specific variable for each group. When user selects an option in one group, the selection in the other group is not affected.
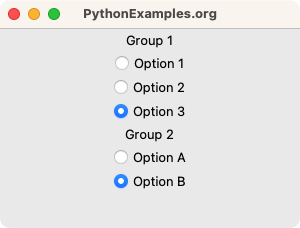
In this tutorial, you will learn how to create two groups of Radiobutton widgets, in a Tk window, with example program.
Example
In this example, we shall create two groups of Radiobutton widget in a Tk window, where there are three options in the first group, and only two options in the second group. We specify a variable group_1_var to the radio buttons in the Group 1, and a variable group_2_var to the radio buttons in the Group 2.
Program
import tkinter as tk
def on_select():
selected_option = radio_var.get()
print(f"Selected option: {selected_option}")
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
# Group 1
group_1_label = tk.Label(window, text="Group 1")
group_1_label.pack()
group_1_var = tk.StringVar()
def group_1_select():
selected_option = group_1_var.get()
print(f"Selected option in Group 1 : {selected_option}")
radio_button_1_1 = tk.Radiobutton(window, text="Option 1", variable=group_1_var, value="Option 1",
command=group_1_select)
radio_button_1_2 = tk.Radiobutton(window, text="Option 2", variable=group_1_var, value="Option 2",
command=group_1_select)
radio_button_1_3 = tk.Radiobutton(window, text="Option 3", variable=group_1_var, value="Option 3",
command=group_1_select)
radio_button_1_1.pack()
radio_button_1_2.pack()
radio_button_1_3.pack()
# Group 2
group_2_label = tk.Label(window, text="Group 2")
group_2_label.pack()
group_2_var = tk.StringVar()
def group_2_select():
selected_option = group_2_var.get()
print(f"Selected option in Group 2 : {selected_option}")
radio_button_2_1 = tk.Radiobutton(window, text="Option A", variable=group_2_var, value="Option 1",
command=group_2_select)
radio_button_2_2 = tk.Radiobutton(window, text="Option B", variable=group_2_var, value="Option 2",
command=group_2_select)
radio_button_2_1.pack()
radio_button_2_2.pack()
# Start the Tkinter event loop
window.mainloop()
Output
Output on MacOS
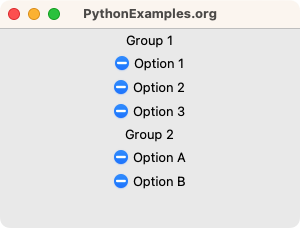
User selects "Option 3" from Group 1 and "Option B" from Group 2.
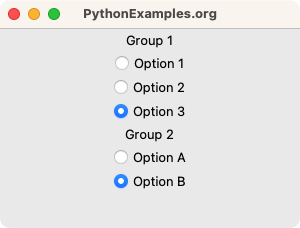
The respective command functions execute, and prints the selected options in the standard output.
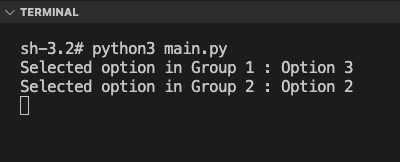
Summary
In this Python Tkinter tutorial, we learned how to create Radiobutton groups and handle the option selection, with examples.