Tkinter Radiobutton – On change of selection
You can set a callback function to execute whenever there is a change in the selection in the Radiobutton group.
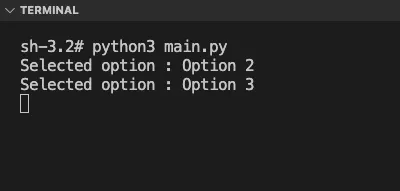
To set a callback function to execute whenever there is a change in the selection in the Radiobutton group in Tkinter, set the command parameter of the Radiobutton widgets with the callback function.
radio_button1 = tk.Radiobutton(window, text="Option 1", variable=radio_var, value="Option 1", command=on_radio_change)
In the above code, the Radiobutton is set with a callback function of on_radio_change that executes whenever this Radiobutton is selected. So, if you give the same callback function for all the Radiobutton widgets in a group, we can track the “on selection change” event of the Radiobutton group.
In this tutorial, you will learn how to set a callback function for the event of selection change in Radiobutton group in Tkinter, with an example program.
Example
In this example, we shall create a group of three Radiobutton widgets with values: Option 1, Option 2, and Option 3. These three Radiobutton widgets are set with on_radio_change function for the command parameter. Whenever there is a change in the selection, this function is called. And in this callback function, we print the newly selected value.
Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
def on_radio_change():
selected_option = radio_var.get()
print(f"Selected option : {selected_option}")
# Create a variable to hold the selected option
radio_var = tk.StringVar()
# Create Radiobuttons
radio_button1 = tk.Radiobutton(window, text="Option 1", variable=radio_var, value="Option 1", command=on_radio_change)
radio_button2 = tk.Radiobutton(window, text="Option 2", variable=radio_var, value="Option 2", command=on_radio_change)
radio_button3 = tk.Radiobutton(window, text="Option 3", variable=radio_var, value="Option 3", command=on_radio_change)
# Pack the Radiobuttons
radio_button1.pack()
radio_button2.pack()
radio_button3.pack()
# Start the Tkinter event loop
window.mainloop()
Output in Windows
Output on MacOS
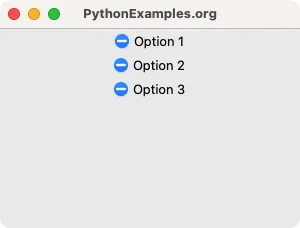
Select Option 2.
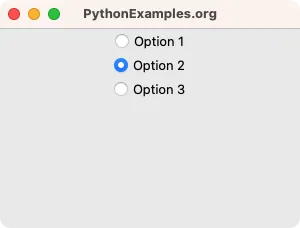
The callback function is called and the selected option is printed to the standard output.
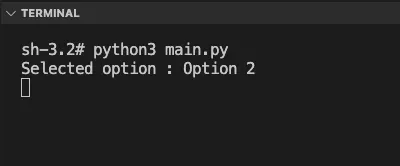
Now, select an other option, say Option 3.
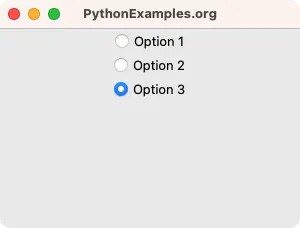
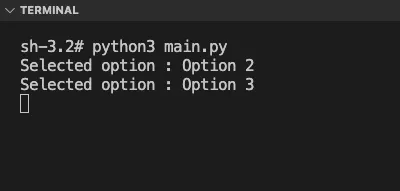
Summary
In this Python Tkinter tutorial, we have seen how to set a callback function to execute whenever there is a change in the selection in Radiobutton group, with examples.