Tkinter Radiobutton – Select an Option
You can select an option from the Radiobutton group programmatically using the variable associated with Radiobutton widgets in the group.
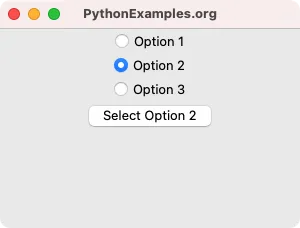
To select an option from the Radiobutton widgets programmatically in Tkinter, set the StringVar associated with the Radiobutton widgets with the value of required option, as shown below.
radio_var.set("Option 2")
In the above code, the Radiobutton with the value Option 2 is selected programmatically.
In this tutorial, you will learn how to select a Radiobutton from the group programmatically in Tkinter, with an example program.
Example
In this example, we shall create a group of three Radiobutton widgets with values: Option 1, Option 2, and Option 3. And also we create a button, which when clicked, selects the Radiobutton with the value Option 2.
Program
import tkinter as tk
# Create the main window
window = tk.Tk()
window.geometry("300x200")
window.title("PythonExamples.org")
# Create a variable to hold the selected option
radio_var = tk.StringVar()
# Create Radiobuttons
radio_button1 = tk.Radiobutton(window, text="Option 1", variable=radio_var, value="Option 1")
radio_button2 = tk.Radiobutton(window, text="Option 2", variable=radio_var, value="Option 2")
radio_button3 = tk.Radiobutton(window, text="Option 3", variable=radio_var, value="Option 3")
# Pack the Radiobuttons
radio_button1.pack()
radio_button2.pack()
radio_button3.pack()
def select_option_2():
radio_var.set("Option 2")
# Button widget
clear_button = tk.Button(window, text="Select Option 2", command=select_option_2)
clear_button.pack()
# Start the Tkinter event loop
window.mainloop()
Output in Windows
Output on MacOS
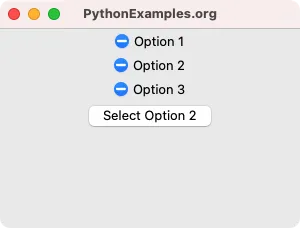
Click on the button Select Option 2. In the event handler function, we select the value Option 2 using the radio_var variable.
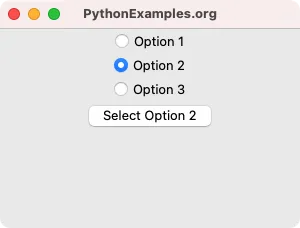
Summary
In this Python Tkinter tutorial, we have seen how to programmatically select a Radiobutton in a group, with examples.